i'm looking for an open source irrlicht scene editor
i'm looking for an open source irrlicht scene editor
hi all,
just want to let you know i'm looking for an open source irrlicht scene editor.
i'm not looking for a full-featured scene editor.
what i basically need is for me to do the following:
1) have access to source code so i can add features to it later.
2) i can add primitive shapes and assign a material to it.
3) i can import a mesh file and assign a material to it.
4) supports model and world transformations.
5) can read/write irr files.
6) has a play button, so i can test the world inside the scene editor.
7) optional: effect file support.
8. optional: lighting for materials with lighting turned on.
it looks similar to mesh viewer, with several added features built-in.
if you have started a project before and are looking for volunteers, i can donate some of my time.
or, if you simply abandoned it, please donate it to me and i'll take care of it.
otherwise, my last resort would be to write one up, which is really not a good idea for me.
just want to let you know i'm looking for an open source irrlicht scene editor.
i'm not looking for a full-featured scene editor.
what i basically need is for me to do the following:
1) have access to source code so i can add features to it later.
2) i can add primitive shapes and assign a material to it.
3) i can import a mesh file and assign a material to it.
4) supports model and world transformations.
5) can read/write irr files.
6) has a play button, so i can test the world inside the scene editor.
7) optional: effect file support.
8. optional: lighting for materials with lighting turned on.
it looks similar to mesh viewer, with several added features built-in.
if you have started a project before and are looking for volunteers, i can donate some of my time.
or, if you simply abandoned it, please donate it to me and i'll take care of it.
otherwise, my last resort would be to write one up, which is really not a good idea for me.
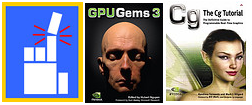
-
- Admin
- Posts: 3590
- Joined: Mon Oct 09, 2006 9:36 am
- Location: Scotland - gonnae no slag aff mah Engleesh
- Contact:
Since I don't know of any open source equivelants of IrrEdit (and since loading/saving .irr files is a requirement) I guess I'll be the first of many to ask:
1) What's wrong with writing plugins to extend IrrEdit?
2) If you really do need source, then why not pay Niko for an IrrEdit source license?
1) What's wrong with writing plugins to extend IrrEdit?
2) If you really do need source, then why not pay Niko for an IrrEdit source license?
Please upload candidate patches to the tracker.
Need help now? IRC to #irrlicht on irc.freenode.net
How To Ask Questions The Smart Way
Need help now? IRC to #irrlicht on irc.freenode.net
How To Ask Questions The Smart Way
-
- Admin
- Posts: 14143
- Joined: Wed Apr 19, 2006 9:20 pm
- Location: Oldenburg(Oldb), Germany
- Contact:
Well, you asked for extensibility of the editor you search. But a plugin-mechanism is not wanted, because you have to write some code? I guess the other editors won't extend by just thinking about extensions. And if you download some of the already existing extensions for irrEdit, you won't need to code anything on your own...
I think the source license costs are heavily realted to whether you will use the editor just for you, or adapt it to in-game usage, if you want to distribute changed versions, if you do a commercial game, ...
I think the source license costs are heavily realted to whether you will use the editor just for you, or adapt it to in-game usage, if you want to distribute changed versions, if you do a commercial game, ...
update on this thread...
i played around with mesh editor and i did manage to add a few code here and there.
it's pretty much a patchwork job, though.
i'm still learning the intricacies of the api, definitely long way to go before i make any decent code.
so, for those who are still interested in a poorman's scene editor, please take this code, play around with it and see if you can add one feature.
just one feature is good enough to get this going.
copy-n-paste it into one of the sample programs and you're set to go.
next feature:
1) arcball for node translation, scaling and rotation.
2) properties menu appear when a node gets a right click event.
i played around with mesh editor and i did manage to add a few code here and there.
it's pretty much a patchwork job, though.
i'm still learning the intricacies of the api, definitely long way to go before i make any decent code.
so, for those who are still interested in a poorman's scene editor, please take this code, play around with it and see if you can add one feature.
just one feature is good enough to get this going.
copy-n-paste it into one of the sample programs and you're set to go.
next feature:
1) arcball for node translation, scaling and rotation.
2) properties menu appear when a node gets a right click event.
Code: Select all
/*
This tutorial show how to create a more complex application with the engine. We construct
a simple mesh viewer using the user interface API and the scenemanagement of Irrlicht.
The tutorial show how to create and use Buttons, Windows, Toolbars, Menus, ComboBoxes,
Tabcontrols, Editboxes, Images, MessageBoxes, SkyBoxes, and how to parse XML files
with the integrated XML reader of the engine.
We start like in most other tutorials: Include all nesessary header files, add a
comment to let the engine be linked with the right .lib file in Visual Studio,
and deklare some global variables. We also add two 'using namespece' statements, so
we do not need to write the whole names of all classes. In this tutorial, we use a
lot stuff from the gui namespace.
*/
#include <irrlicht.h>
#include <iostream>
using namespace irr;
using namespace gui;
#pragma comment(lib, "Irrlicht.lib")
IrrlichtDevice *Device = 0;
core::stringc StartUpModelFile;
core::stringw MessageText;
core::stringw Caption;
scene::IAnimatedMeshSceneNode* Model = 0;
scene::ISceneNode* SkyBox = 0;
scene::ICameraSceneNode* Camera[2] = { 0, 0};
/*
toggles between various cameras
*/
void setActiveCamera ( scene::ICameraSceneNode* newActive )
{
if ( 0 == Device )
return;
scene::ICameraSceneNode* active = Device->getSceneManager()->getActiveCamera ();
newActive->setInputReceiverEnabled ( true );
Device->getSceneManager()->setActiveCamera ( newActive );
}
/*
The three following functions do several stuff used by the mesh viewer.
The first function showAboutText() simply displays a messagebox with a caption
and a message text. The texts will be stored in the MessageText and
Caption variables at startup.
*/
void showAboutText()
{
// create modal message box with the text
// loaded from the xml file.
Device->getGUIEnvironment()->addMessageBox(
Caption.c_str(), MessageText.c_str());
}
/*
The second function loadModel() loads a model and displays it using an
addAnimatedMeshSceneNode and the scene manager. Nothing difficult. It also
displays a short message box, if the model could not be loaded.
*/
void loadModel(const c8* fn)
{
// modify the name if it a .pk3 file
core::stringc filename ( fn );
core::stringc extension;
core::getFileNameExtension ( extension, filename );
extension.make_lower();
// if a texture is loaded apply it to the current model..
if ( extension == ".jpg" ||
extension == ".pcx" ||
extension == ".png" ||
extension == ".ppm" ||
extension == ".pgm" ||
extension == ".pbm" ||
extension == ".psd" ||
extension == ".tga" ||
extension == ".bmp"
)
{
video::ITexture * texture = Device->getVideoDriver()->getTexture( filename.c_str() );
if ( texture && Model )
{
// always reload texture
Device->getVideoDriver()->removeTexture ( texture );
texture = Device->getVideoDriver()->getTexture( filename.c_str() );
Model->setMaterialTexture ( 0, texture );
}
return;
}
// if a archive is loaded add it to the FileSystems..
if ( extension == ".pk3" ||
extension == ".zip"
)
{
Device->getFileSystem()->addZipFileArchive( filename.c_str () );
return;
}
// load a model into the engine
if (Model)
Model->remove();
Model = 0;
scene::IAnimatedMesh* m = Device->getSceneManager()->getMesh( filename.c_str() );
if (!m)
{
// model could not be loaded
if (StartUpModelFile != filename)
Device->getGUIEnvironment()->addMessageBox(
Caption.c_str(), L"The model could not be loaded. " \
L"Maybe it is not a supported file format.");
return;
}
// set default material properties
Model = Device->getSceneManager()->addAnimatedMeshSceneNode(m);
Model->setMaterialFlag(video::EMF_LIGHTING, false);
// Model->setMaterialFlag(video::EMF_BACK_FACE_CULLING, false);
Model->setDebugDataVisible(scene::EDS_OFF);
Model->setAnimationSpeed(30);
}
/*
Finally, the third function creates a toolbox window. In this simple mesh viewer,
this toolbox only contains a tab control with three edit boxes for changing
the scale of the displayed model.
*/
void createToolBox()
{
// remove tool box if already there
IGUIEnvironment* env = Device->getGUIEnvironment();
IGUIElement* root = env->getRootGUIElement();
IGUIElement* e = root->getElementFromId(5000, true);
if (e) e->remove();
// create the toolbox window
IGUIWindow* wnd = env->addWindow(core::rect<s32>(600,25,800,480),
false, L"Toolset", 0, 5000);
// create tab control and tabs
IGUITabControl* tab = env->addTabControl(
core::rect<s32>(2,20,800-602,480-7), wnd, true, true);
IGUITab* t1 = tab->addTab(L"Scale");
// add some edit boxes and a button to tab one
env->addEditBox(L"1.0", core::rect<s32>(40,50,130,70), true, t1, 901);
env->addEditBox(L"1.0", core::rect<s32>(40,80,130,100), true, t1, 902);
env->addEditBox(L"1.0", core::rect<s32>(40,110,130,130), true, t1, 903);
env->addButton(core::rect<s32>(10,150,100,190), t1, 1101, L"set");
// add senseless checkbox
env->addCheckBox(true, core::rect<s32>(10,220,200,240), t1, -1, L"Senseless Checkbox");
// add undocumentated transparent control
env->addStaticText(L"Transparent Control:", core::rect<s32>(10,240,150,260), true, false, t1);
IGUIScrollBar* scrollbar = env->addScrollBar(true, core::rect<s32>(10,260,150,275), t1, 104);
scrollbar->setMax(255);
scrollbar->setPos(255);
// bring irrlicht engine logo to front, because it
// now may be below the newly created toolbox
root->bringToFront(root->getElementFromId(666, true));
}
/*
To get all the events sent by the GUI Elements, we need to create an event
receiver. This one is really simple. If an event occurs, it checks the id
of the caller and the event type, and starts an action based on these values.
For example, if a menu item with id 100 was selected, if opens a file-open-dialog.
*/
class MyEventReceiver : public IEventReceiver
{
public:
virtual bool OnEvent(const SEvent& event)
{
if (Camera[0] != 0 && event.EventType == irr::EET_KEY_INPUT_EVENT&&
event.KeyInput.PressedDown)
{
core::vector3df pos = Camera[0]->getPosition();
switch(event.KeyInput.Key)
{
case KEY_KEY_A:
{
pos.X += 2.0f;
}
break;
case KEY_KEY_D:
{
pos.X += -2.0f;
}
break;
case KEY_KEY_W:
{
pos.Y += 2.0f;
}
break;
case KEY_KEY_S:
{
pos.Y += -2.0f;
}
break;
}
Camera[0]->setPosition(pos);
}
// Escape swaps Camera Input
if (event.EventType == EET_KEY_INPUT_EVENT &&
event.KeyInput.Key == irr::KEY_ESCAPE &&
event.KeyInput.PressedDown == false)
{
if ( Device )
{
scene::ICameraSceneNode * camera = Device->getSceneManager()->getActiveCamera ();
if ( camera )
{
camera->setInputReceiverEnabled ( !camera->isInputReceiverEnabled() );
}
return true;
}
}
if ( event.EventType == EET_MOUSE_INPUT_EVENT ) {
switch(event.MouseInput.Event)
{
case EMIE_MOUSE_WHEEL:
{
}
break;
case EMIE_LMOUSE_PRESSED_DOWN:
{
printf("EMIE_LMOUSE_PRESSED_DOWN\n");
core::position2di p = core::position2di(event.MouseInput.X,event.MouseInput.Y);
printf("X=%d\n", p.X);
printf("Y=%d\n", p.Y);
scene::ISceneCollisionManager *icm = Device->getSceneManager()->getSceneCollisionManager();
scene::ICameraSceneNode *cam = Device->getSceneManager()->getActiveCamera();
//core::line3d<f32> ray = icm->getRayFromScreenCoordinates(core::position2d<s32>(p.X,p.Y),cam);
scene::ISceneNode *selNode = icm->getSceneNodeFromScreenCoordinatesBB(core::position2d<s32>(p.X,p.Y),0,false);
selNode->setScale(core::vector3df(0.5,0.5,0.5));
}
break;
}
}
if (event.EventType == EET_GUI_EVENT)
{
s32 id = event.GUIEvent.Caller->getID();
IGUIEnvironment* env = Device->getGUIEnvironment();
switch(event.GUIEvent.EventType)
{
case EGET_MENU_ITEM_SELECTED:
{
// a menu item was clicked
IGUIContextMenu* menu = (IGUIContextMenu*)event.GUIEvent.Caller;
s32 id = menu->getItemCommandId(menu->getSelectedItem());
switch(id)
{
case 100: // File -> Open Model
env->addFileOpenDialog(L"Please select a model file to open");
break;
case 101: // File -> Set Model Archive
env->addFileOpenDialog(L"Please select your game archive/directory");
break;
case 200: // File -> Quit
Device->closeDevice();
break;
case 300: // View -> Skybox
SkyBox->setVisible(!SkyBox->isVisible());
break;
case 400: // View -> Debug Information
if (Model)
Model->setDebugDataVisible(scene::EDS_OFF);
break;
case 410: // View -> Debug Information
if (Model)
Model->setDebugDataVisible((scene::E_DEBUG_SCENE_TYPE)(Model->isDebugDataVisible()^scene::EDS_BBOX));
break;
case 420: // View -> Debug Information
if (Model)
Model->setDebugDataVisible((scene::E_DEBUG_SCENE_TYPE)(Model->isDebugDataVisible()^scene::EDS_NORMALS));
break;
case 430: // View -> Debug Information
if (Model)
Model->setDebugDataVisible((scene::E_DEBUG_SCENE_TYPE)(Model->isDebugDataVisible()^scene::EDS_SKELETON));
break;
case 440: // View -> Debug Information
if (Model)
Model->setDebugDataVisible((scene::E_DEBUG_SCENE_TYPE)(Model->isDebugDataVisible()^scene::EDS_MESH_WIRE_OVERLAY));
break;
case 450: // View -> Debug Information
if (Model)
Model->setDebugDataVisible((scene::E_DEBUG_SCENE_TYPE)(Model->isDebugDataVisible()^scene::EDS_HALF_TRANSPARENCY));
break;
case 460: // View -> Debug Information
if (Model)
Model->setDebugDataVisible((scene::E_DEBUG_SCENE_TYPE)(Model->isDebugDataVisible()^scene::EDS_BBOX_BUFFERS));
break;
case 499: // View -> Debug Information
if (Model)
Model->setDebugDataVisible(scene::EDS_FULL);
break;
case 500: // Help->About
showAboutText();
break;
case 610: // View -> Material -> Solid
if (Model)
Model->setMaterialType(video::EMT_SOLID);
break;
case 620: // View -> Material -> Transparent
if (Model)
Model->setMaterialType(video::EMT_TRANSPARENT_ADD_COLOR);
break;
case 630: // View -> Material -> Reflection
if (Model)
Model->setMaterialType(video::EMT_SPHERE_MAP);
break;
case 1000:
setActiveCamera ( Camera[0] );
break;
case 1100:
setActiveCamera ( Camera[1] );
break;
case 5001:
printf("Add Sphere\n");
scene::ISceneNode *n1 = Device->getSceneManager()->addSphereSceneNode();
n1->setScale(core::vector3df(2,2,2));
break;
}
break;
}
case EGET_FILE_SELECTED:
{
// load the model file, selected in the file open dialog
IGUIFileOpenDialog* dialog =
(IGUIFileOpenDialog*)event.GUIEvent.Caller;
loadModel(core::stringc(dialog->getFileName()).c_str());
}
case EGET_SCROLL_BAR_CHANGED:
// control skin transparency
if (id == 104)
{
s32 pos = ((IGUIScrollBar*)event.GUIEvent.Caller)->getPos();
for (s32 i=0; i<irr::gui::EGDC_COUNT ; ++i)
{
video::SColor col = env->getSkin()->getColor((EGUI_DEFAULT_COLOR)i);
col.setAlpha(pos);
env->getSkin()->setColor((EGUI_DEFAULT_COLOR)i, col);
}
}
break;
case EGET_COMBO_BOX_CHANGED:
// control anti-aliasing/filtering
if (id == 108)
{
s32 pos = ((IGUIComboBox*)event.GUIEvent.Caller)->getSelected();
switch (pos)
{
case 0:
if (Model)
{
Model->setMaterialFlag(video::EMF_BILINEAR_FILTER, false);
Model->setMaterialFlag(video::EMF_TRILINEAR_FILTER, false);
Model->setMaterialFlag(video::EMF_ANISOTROPIC_FILTER, false);
}
break;
case 1:
if (Model)
{
Model->setMaterialFlag(video::EMF_BILINEAR_FILTER, true);
Model->setMaterialFlag(video::EMF_TRILINEAR_FILTER, false);
}
break;
case 2:
if (Model)
{
Model->setMaterialFlag(video::EMF_BILINEAR_FILTER, false);
Model->setMaterialFlag(video::EMF_TRILINEAR_FILTER, true);
}
break;
case 3:
if (Model)
{
Model->setMaterialFlag(video::EMF_ANISOTROPIC_FILTER, true);
}
break;
case 4:
if (Model)
{
Model->setMaterialFlag(video::EMF_ANISOTROPIC_FILTER, false);
}
break;
}
}
break;
case EGET_BUTTON_CLICKED:
switch(id)
{
case 1101:
{
// set scale
gui::IGUIElement* root = env->getRootGUIElement();
core::vector3df scale;
core::stringc s;
s = root->getElementFromId(901, true)->getText();
scale.X = (f32)atof(s.c_str());
s = root->getElementFromId(902, true)->getText();
scale.Y = (f32)atof(s.c_str());
s = root->getElementFromId(903, true)->getText();
scale.Z = (f32)atof(s.c_str());
if (Model)
Model->setScale(scale);
}
break;
case 1102:
env->addFileOpenDialog(L"Please select a model file to open");
break;
case 1103:
showAboutText();
break;
case 1104:
createToolBox();
break;
case 1105:
env->addFileOpenDialog(L"Please select your game archive/directory");
break;
}
break;
}
}
return false;
}
};
/*
Most of the hard work is done. We only need to create the Irrlicht Engine device
and all the buttons, menus and toolbars.
We start up the engine as usual, using createDevice(). To make our application
catch events, we set our eventreceiver as parameter. The #ifdef WIN32 preprocessor
commands are not necesarry, but I included them to make the tutorial use DirectX on
Windows and OpenGL on all other platforms like Linux.
As you can see, there is also a unusual call to IrrlichtDevice::setResizeAble().
This makes the render window resizeable, which is quite useful for a mesh viewer.
*/
int main()
{
// ask user for driver
video::E_DRIVER_TYPE driverType = video::EDT_DIRECT3D8;
printf("Please select the driver you want for this example:\n"\
" (a) Direct3D 9.0c\n (b) Direct3D 8.1\n (c) OpenGL 1.5\n"\
" (d) Software Renderer\n (e) Burning's Software Renderer\n"\
" (f) NullDevice\n (otherKey) exit\n\n");
char key;
//std::cin >> key;
key ='a';
switch(key)
{
case 'a': driverType = video::EDT_DIRECT3D9;break;
case 'b': driverType = video::EDT_DIRECT3D8;break;
case 'c': driverType = video::EDT_OPENGL; break;
case 'd': driverType = video::EDT_SOFTWARE; break;
case 'e': driverType = video::EDT_BURNINGSVIDEO;break;
case 'f': driverType = video::EDT_NULL; break;
default: return 1;
}
// create device and exit if creation failed
MyEventReceiver receiver;
Device = createDevice(driverType, core::dimension2d<s32>(1024, 768),
16, false, false, false, &receiver);
if (Device == 0)
return 1; // could not create selected driver.
Device->setResizeAble(true);
Device->setWindowCaption(L"Irrlicht Engine - Loading...");
video::IVideoDriver* driver = Device->getVideoDriver();
IGUIEnvironment* env = Device->getGUIEnvironment();
scene::ISceneManager* smgr = Device->getSceneManager();
smgr->getParameters()->setAttribute(scene::COLLADA_CREATE_SCENE_INSTANCES, true);
driver->setTextureCreationFlag(video::ETCF_ALWAYS_32_BIT, true);
smgr->addLightSceneNode();
smgr->addLightSceneNode(0, core::vector3df(50,-50,100), video::SColorf(1.0f,1.0f,1.0f),20000);
// add our media directory as "search path"
Device->getFileSystem()->addFolderFileArchive ( "../../media/" );
/*
The next step is to read the configuration file. It is stored in the xml
format and looks a little bit like this:
<?xml version="1.0"?>
<config>
<startUpModel file="some filename" />
<messageText caption="Irrlicht Engine Mesh Viewer">
Hello!
</messageText>
</config>
We need the data stored in there to be written into the global variables
StartUpModelFile, MessageText and Caption. This is now done using the
Irrlicht Engine integrated XML parser:
*/
// read configuration from xml file
io::IXMLReader* xml = Device->getFileSystem()->createXMLReader(
"config.xml");
while(xml && xml->read())
{
switch(xml->getNodeType())
{
case io::EXN_TEXT:
// in this xml file, the only text which occurs is the messageText
MessageText = xml->getNodeData();
break;
case io::EXN_ELEMENT:
{
if (core::stringw("startUpModel") == xml->getNodeName())
StartUpModelFile = xml->getAttributeValue(L"file");
else
if (core::stringw("messageText") == xml->getNodeName())
Caption = xml->getAttributeValue(L"caption");
}
break;
}
}
if (xml)
xml->drop(); // don't forget to delete the xml reader
/*
That wasn't difficult. Now we'll set a nicer font and create the
Menu. It is possible to create submenus for every menu item. The call
menu->addItem(L"File", -1, true, true); for example adds a new menu
Item with the name "File" and the id -1. The following parameter says
that the menu item should be enabled, and the last one says, that
there should be a submenu. The submenu can now be accessed with
menu->getSubMenu(0), because the "File" entry is the menu item with
index 0.
*/
// set a nicer font
IGUISkin* skin = env->getSkin();
IGUIFont* font = env->getFont("fonthaettenschweiler.bmp");
if (font)
skin->setFont(font);
// create menu
gui::IGUIContextMenu* menu = env->addMenu();
menu->addItem(L"File", -1, true, true);
menu->addItem(L"View", -1, true, true);
menu->addItem(L"Camera", -1, true, true);
menu->addItem(L"Help", -1, true, true);
menu->addItem(L"Insert", -1, true, true);
gui::IGUIContextMenu* submenu;
submenu = menu->getSubMenu(0);
submenu->addItem(L"Open Model File & Texture...", 100);
submenu->addItem(L"Set Model Archive...", 101);
submenu->addSeparator();
submenu->addItem(L"Quit", 200);
submenu = menu->getSubMenu(1);
submenu->addItem(L"toggle sky box visibility", 300);
submenu->addItem(L"toggle model debug information", -1, true, true);
submenu->addItem(L"model material", -1, true, true );
submenu = submenu->getSubMenu(1);
submenu->addItem(L"Off", 400);
submenu->addItem(L"Bounding Box", 410);
submenu->addItem(L"Normals", 420);
submenu->addItem(L"Skeleton", 430);
submenu->addItem(L"Wire overlay", 440);
submenu->addItem(L"Half-Transparent", 450);
submenu->addItem(L"Buffers bounding boxes", 460);
submenu->addItem(L"All", 499);
submenu = menu->getSubMenu(1)->getSubMenu(2);
submenu->addItem(L"Solid", 610);
submenu->addItem(L"Transparent", 620);
submenu->addItem(L"Reflection", 630);
submenu = menu->getSubMenu(2);
submenu->addItem(L"Maya Style", 1000);
submenu->addItem(L"First Person", 1100);
submenu = menu->getSubMenu(3);
submenu->addItem(L"About", 500);
submenu = menu->getSubMenu(4);
submenu->addItem(L"Sphere", 5001);
/*
Below the toolbar, we want a toolbar, onto which we can place
colored buttons and important looking stuff like a senseless
combobox.
*/
// create toolbar
gui::IGUIToolBar* bar = env->addToolBar();
video::ITexture* image = driver->getTexture("open.png");
bar->addButton(1102, 0, L"Open a model",image, 0, false, true);
image = driver->getTexture("tools.png");
bar->addButton(1104, 0, L"Open Toolset",image, 0, false, true);
image = driver->getTexture("zip.png");
bar->addButton(1105, 0, L"Set Model Archive",image, 0, false, true);
image = driver->getTexture("help.png");
bar->addButton(1103, 0, L"Open Help", image, 0, false, true);
// create a combobox with some senseless texts
gui::IGUIComboBox* box = env->addComboBox(core::rect<s32>(250,4,350,23), bar, 108);
box->addItem(L"No filtering");
box->addItem(L"Bilinear");
box->addItem(L"Trilinear");
box->addItem(L"Anisotropic");
box->addItem(L"Isotropic");
/*
To make the editor look a little bit better, we disable transparent
gui elements, and add a Irrlicht Engine logo. In addition, a text
showing the current frame per second value is created and
the window caption is changed.
*/
// disable alpha
for (s32 i=0; i<gui::EGDC_COUNT ; ++i)
{
video::SColor col = env->getSkin()->getColor((gui::EGUI_DEFAULT_COLOR)i);
col.setAlpha(255);
env->getSkin()->setColor((gui::EGUI_DEFAULT_COLOR)i, col);
}
// add a tabcontrol
createToolBox();
// create fps text
IGUIStaticText* fpstext = env->addStaticText(L"", core::rect<s32>(400,4,570,23), true, false, bar);
// set window caption
Caption += " - [";
Caption += driver->getName();
Caption += "]";
Device->setWindowCaption(Caption.c_str());
/*
That's nearly the whole application. We simply show the about
message box at start up, and load the first model. To make everything
look better, a skybox is created and a user controled camera,
to make the application a little bit more interactive. Finally,
everything is drawed in a standard drawing loop.
*/
// show about message box and load default model
showAboutText();
loadModel(StartUpModelFile.c_str());
// add skybox
SkyBox = smgr->addSkyBoxSceneNode(
driver->getTexture("irrlicht2_up.jpg"),
driver->getTexture("irrlicht2_dn.jpg"),
driver->getTexture("irrlicht2_lf.jpg"),
driver->getTexture("irrlicht2_rt.jpg"),
driver->getTexture("irrlicht2_ft.jpg"),
driver->getTexture("irrlicht2_bk.jpg"));
// add a camera scene node
Camera[0] = smgr->addCameraSceneNodeMaya();
Camera[1] = smgr->addCameraSceneNodeFPS();
setActiveCamera ( Camera[0] );
scene::ITriangleSelector* selector = 0;
Camera[0]->setTriangleSelector(selector);
Camera[1]->setTriangleSelector(selector);
// load the irrlicht engine logo
IGUIImage *img =
env->addImage(driver->getTexture("irrlichtlogo2.png"),
core::position2d<s32>(10, driver->getScreenSize().Height - 128));
// lock the logo's edges to the bottom left corner of the screen
img->setAlignment(EGUIA_UPPERLEFT, EGUIA_UPPERLEFT, EGUIA_LOWERRIGHT, EGUIA_LOWERRIGHT);
// draw everything
while(Device->run() && driver)
{
if (Device->isWindowActive())
{
driver->beginScene(true, true, video::SColor(150,50,50,50));
smgr->drawAll();
env->drawAll();
driver->endScene();
core::stringw str(L"FPS: ");
str.append(core::stringw(driver->getFPS()));
str += L" Tris: ";
str.append(core::stringw(driver->getPrimitiveCountDrawn()));
fpstext->setText(str.c_str());
}
else
Device->yield();
}
Device->drop();
return 0;
}
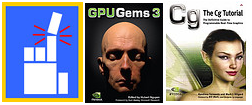
i know what u mean...but that's not what i'm looking for.hybrid wrote:Well, you asked for extensibility of the editor you search. But a plugin-mechanism is not wanted, because you have to write some code? I guess the other editors won't extend by just thinking about extensions. And if you download some of the already existing extensions for irrEdit, you won't need to code anything on your own...
I think the source license costs are heavily realted to whether you will use the editor just for you, or adapt it to in-game usage, if you want to distribute changed versions, if you do a commercial game, ...
i'm sorry if this deal will not work out.
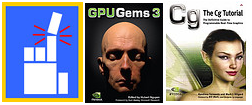
I never heard about working open source Irrlicht editor. There are several attempts, bur all was abandoned.
You can write your own editor, but must spend approximately 2 years of your sparing time and more. My own project was released after 2 years hard work. You can see how much time has been spends Nico's IrrEdit at this stage of development.
Your simple requirements - 1.2.3.... are not that much for implementation. In this case you must create any object inspector that catches Irrlicht sterilization. But then you will get universal Irrlicht Property Inspector. Yet I thinks that if your are experienced programmer will never occur any big difficulty in this scope.
Tomorrow will upload new 1.0 version of VisualEditor and will inform about this in correspondent thread when finish with web site. After approximately two years frozen period
But it's not open source. If you like it just use the free version.
You can write your own editor, but must spend approximately 2 years of your sparing time and more. My own project was released after 2 years hard work. You can see how much time has been spends Nico's IrrEdit at this stage of development.
Your simple requirements - 1.2.3.... are not that much for implementation. In this case you must create any object inspector that catches Irrlicht sterilization. But then you will get universal Irrlicht Property Inspector. Yet I thinks that if your are experienced programmer will never occur any big difficulty in this scope.
Tomorrow will upload new 1.0 version of VisualEditor and will inform about this in correspondent thread when finish with web site. After approximately two years frozen period
But it's not open source. If you like it just use the free version.

i just got the transform handles coded, see image below for details. i used a billboard to show the position of the handle.
i used a simple 3dline drawing function for now, a node will replace each axis later. an arcball would be nice for rotation.

i used a simple 3dline drawing function for now, a node will replace each axis later. an arcball would be nice for rotation.

Code: Select all
if ( selNode > 0 ) {
core::vector3df p = selNode->getAbsolutePosition();
//printf("X=%d\n", p.X);
//printf("Y=%d\n", p.Y);
//printf("Z=%d\n", p.Z);
bill->setPosition(p);
core::vector3df p1(p.X+40, p.Y, p.Z);
core::vector3df p2(p.X, p.Y+40, p.Z);
core::vector3df p3(p.X, p.Y, p.Z+40);
driver->setTransform(video::ETS_WORLD, core::matrix4());
driver->setMaterial(material);
driver->draw3DLine(p,p1,video::SColor(255,0,0,255));
driver->draw3DLine(p,p2,video::SColor(255,0,255,0));
driver->draw3DLine(p,p3,video::SColor(255,255,0,0));
}
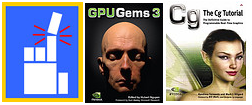
you are very right on that one, etcaptor. i do agree every single word you wrote.etcaptor wrote:I never heard about working open source Irrlicht editor. There are several attempts, bur all was abandoned.
You can write your own editor, but must spend approximately 2 years of your sparing time and more. My own project was released after 2 years hard work. You can see how much time has been spends Nico's IrrEdit at this stage of development.
Your simple requirements - 1.2.3.... are not that much for implementation. In this case you must create any object inspector that catches Irrlicht sterilization. But then you will get universal Irrlicht Property Inspector. Yet I thinks that if your are experienced programmer will never occur any big difficulty in this scope.
Tomorrow will upload new 1.0 version of VisualEditor and will inform about this in correspondent thread when finish with web site. After approximately two years frozen period
But it's not open source. If you like it just use the free version.
i've seen your product and it looks pretty good. looks really awesome.
but then again, it doesn't fit my requirements. nor align to our use cases here. really sorry to say that, though.
plus, this tool has zero commercial value to me, so it's better to give it away so anyone can take it and add code to it.
two years is really hard work, i'm sure the amount of hours that went into it is big. my simple tool won't compete with yours, because my tool is going to be so simple only it will look pathetic sitting beside your cool app.
i'm hoping to include nadro's code along with other free code posted here. maybe i could get away with something cool and be able to put a shader module in there later.
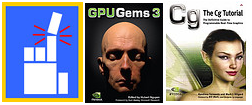
here's the code.
copy and paste the code in one of the tutorials, for example the mesh viewer tutorial. then build and run, should be able to see the output.
copy and paste the code in one of the tutorials, for example the mesh viewer tutorial. then build and run, should be able to see the output.
Code: Select all
/*
This tutorial show how to create a more complex application with the engine. We construct
a simple mesh viewer using the user interface API and the scenemanagement of Irrlicht.
The tutorial show how to create and use Buttons, Windows, Toolbars, Menus, ComboBoxes,
Tabcontrols, Editboxes, Images, MessageBoxes, SkyBoxes, and how to parse XML files
with the integrated XML reader of the engine.
We start like in most other tutorials: Include all nesessary header files, add a
comment to let the engine be linked with the right .lib file in Visual Studio,
and deklare some global variables. We also add two 'using namespece' statements, so
we do not need to write the whole names of all classes. In this tutorial, we use a
lot stuff from the gui namespace.
*/
#include <irrlicht.h>
#include <iostream>
using namespace irr;
using namespace gui;
using namespace video;
using namespace scene;
#pragma comment(lib, "Irrlicht.lib")
IrrlichtDevice *Device = 0;
core::stringc StartUpModelFile;
core::stringw MessageText;
core::stringw Caption;
scene::IAnimatedMeshSceneNode* Model = 0;
scene::ISceneNode* SkyBox = 0;
scene::ISceneNode *selNode = 0;
scene::ICameraSceneNode* Camera[2] = { 0, 0};
/*
toggles between various cameras
*/
void setActiveCamera ( scene::ICameraSceneNode* newActive )
{
if ( 0 == Device )
return;
scene::ICameraSceneNode* active = Device->getSceneManager()->getActiveCamera ();
newActive->setInputReceiverEnabled ( true );
Device->getSceneManager()->setActiveCamera ( newActive );
}
/*
The three following functions do several stuff used by the mesh viewer.
The first function showAboutText() simply displays a messagebox with a caption
and a message text. The texts will be stored in the MessageText and
Caption variables at startup.
*/
void showAboutText()
{
// create modal message box with the text
// loaded from the xml file.
Device->getGUIEnvironment()->addMessageBox(
Caption.c_str(), MessageText.c_str());
}
/*
The second function loadModel() loads a model and displays it using an
addAnimatedMeshSceneNode and the scene manager. Nothing difficult. It also
displays a short message box, if the model could not be loaded.
*/
void loadModel(const c8* fn)
{
// modify the name if it a .pk3 file
core::stringc filename ( fn );
core::stringc extension;
core::getFileNameExtension ( extension, filename );
extension.make_lower();
// if a texture is loaded apply it to the current model..
if ( extension == ".jpg" ||
extension == ".pcx" ||
extension == ".png" ||
extension == ".ppm" ||
extension == ".pgm" ||
extension == ".pbm" ||
extension == ".psd" ||
extension == ".tga" ||
extension == ".bmp"
)
{
video::ITexture * texture = Device->getVideoDriver()->getTexture( filename.c_str() );
if ( texture && Model )
{
// always reload texture
Device->getVideoDriver()->removeTexture ( texture );
texture = Device->getVideoDriver()->getTexture( filename.c_str() );
Model->setMaterialTexture ( 0, texture );
}
return;
}
// if a archive is loaded add it to the FileSystems..
if ( extension == ".pk3" ||
extension == ".zip"
)
{
Device->getFileSystem()->addZipFileArchive( filename.c_str () );
return;
}
// load a model into the engine
//if (Model)
// Model->remove();
//Model = 0;
scene::IAnimatedMesh* m = Device->getSceneManager()->getMesh( filename.c_str() );
if (!m)
{
// model could not be loaded
if (StartUpModelFile != filename)
Device->getGUIEnvironment()->addMessageBox(
Caption.c_str(), L"The model could not be loaded. " \
L"Maybe it is not a supported file format.");
return;
}
// set default material properties
scene::ISceneNode* sceneRoot = Device->getSceneManager()->getRootSceneNode();
Model = Device->getSceneManager()->addAnimatedMeshSceneNode(m,sceneRoot);
Model->setMaterialFlag(video::EMF_LIGHTING, false);
// Model->setMaterialFlag(video::EMF_BACK_FACE_CULLING, false);
Model->setDebugDataVisible(scene::EDS_OFF);
Model->setAnimationSpeed(30);
selNode = Model;
}
/*
Finally, the third function creates a toolbox window. In this simple mesh viewer,
this toolbox only contains a tab control with three edit boxes for changing
the scale of the displayed model.
*/
void createToolBox()
{
// remove tool box if already there
IGUIEnvironment* env = Device->getGUIEnvironment();
IGUIElement* root = env->getRootGUIElement();
IGUIElement* e = root->getElementFromId(5000, true);
if (e) e->remove();
// create the toolbox window
IGUIWindow* wnd = env->addWindow(core::rect<s32>(800,25,1000,480),
false, L"Toolset", 0, 5000);
// create tab control and tabs
IGUITabControl* tab = env->addTabControl(
core::rect<s32>(2,20,800-602,480-7), wnd, true, true);
IGUITab* t1 = tab->addTab(L"Scale");
// add some edit boxes and a button to tab one
env->addEditBox(L"1.0", core::rect<s32>(40,50,130,70), true, t1, 901);
env->addEditBox(L"1.0", core::rect<s32>(40,80,130,100), true, t1, 902);
env->addEditBox(L"1.0", core::rect<s32>(40,110,130,130), true, t1, 903);
env->addButton(core::rect<s32>(10,150,100,190), t1, 1101, L"set");
// add senseless checkbox
env->addCheckBox(true, core::rect<s32>(10,220,200,240), t1, -1, L"Senseless Checkbox");
// add undocumentated transparent control
env->addStaticText(L"Transparent Control:", core::rect<s32>(10,240,150,260), true, false, t1);
IGUITab* t2 = tab->addTab(L"Translate");
// add some edit boxes and a button to tab one
env->addEditBox(L"1.0", core::rect<s32>(40,50,130,70), true, t2, 904);
env->addEditBox(L"1.0", core::rect<s32>(40,80,130,100), true, t2, 905);
env->addEditBox(L"1.0", core::rect<s32>(40,110,130,130), true, t2, 906);
env->addButton(core::rect<s32>(10,150,100,190), t2, 11010, L"set");
IGUITab* t3 = tab->addTab(L"Rotate");
// add some edit boxes and a button to tab one
env->addEditBox(L"1.0", core::rect<s32>(40,50,130,70), true, t3, 907);
env->addEditBox(L"1.0", core::rect<s32>(40,80,130,100), true, t3, 908);
env->addEditBox(L"1.0", core::rect<s32>(40,110,130,130), true, t3, 909);
env->addButton(core::rect<s32>(10,150,100,190), t3, 11011, L"set");
IGUIScrollBar* scrollbar = env->addScrollBar(true, core::rect<s32>(10,260,150,275), t1, 104);
scrollbar->setMax(255);
scrollbar->setPos(255);
// bring irrlicht engine logo to front, because it
// now may be below the newly created toolbox
root->bringToFront(root->getElementFromId(666, true));
}
/*
To get all the events sent by the GUI Elements, we need to create an event
receiver. This one is really simple. If an event occurs, it checks the id
of the caller and the event type, and starts an action based on these values.
For example, if a menu item with id 100 was selected, if opens a file-open-dialog.
*/
class MyEventReceiver : public IEventReceiver
{
public:
virtual bool OnEvent(const SEvent& event)
{
if (Camera[0] != 0 && event.EventType == irr::EET_KEY_INPUT_EVENT&&
event.KeyInput.PressedDown)
{
core::vector3df pos = Camera[0]->getPosition();
switch(event.KeyInput.Key)
{
case KEY_KEY_A:
{
pos.X += 2.0f;
}
break;
case KEY_KEY_D:
{
pos.X += -2.0f;
}
break;
case KEY_KEY_W:
{
pos.Y += 2.0f;
}
break;
case KEY_KEY_S:
{
pos.Y += -2.0f;
}
break;
}
Camera[0]->setPosition(pos);
}
// Escape swaps Camera Input
if (event.EventType == EET_KEY_INPUT_EVENT &&
event.KeyInput.Key == irr::KEY_ESCAPE &&
event.KeyInput.PressedDown == false)
{
if ( Device )
{
scene::ICameraSceneNode * camera = Device->getSceneManager()->getActiveCamera ();
if ( camera )
{
camera->setInputReceiverEnabled ( !camera->isInputReceiverEnabled() );
}
return true;
}
}
if (event.EventType == EET_GUI_EVENT)
{
s32 id = event.GUIEvent.Caller->getID();
IGUIEnvironment* env = Device->getGUIEnvironment();
switch(event.GUIEvent.EventType)
{
case EGET_MENU_ITEM_SELECTED:
{
// a menu item was clicked
IGUIContextMenu* menu = (IGUIContextMenu*)event.GUIEvent.Caller;
s32 id = menu->getItemCommandId(menu->getSelectedItem());
switch(id)
{
case 100: // File -> Open Model
env->addFileOpenDialog(L"Please select a model file to open");
break;
case 101: // File -> Set Model Archive
env->addFileOpenDialog(L"Please select your game archive/directory");
break;
case 200: // File -> Quit
Device->closeDevice();
break;
case 300: // View -> Skybox
SkyBox->setVisible(!SkyBox->isVisible());
break;
case 400: // View -> Debug Information
if (Model)
Model->setDebugDataVisible(scene::EDS_OFF);
break;
case 410: // View -> Debug Information
if (Model)
Model->setDebugDataVisible((scene::E_DEBUG_SCENE_TYPE)(Model->isDebugDataVisible()^scene::EDS_BBOX));
break;
case 420: // View -> Debug Information
if (Model)
Model->setDebugDataVisible((scene::E_DEBUG_SCENE_TYPE)(Model->isDebugDataVisible()^scene::EDS_NORMALS));
break;
case 430: // View -> Debug Information
if (Model)
Model->setDebugDataVisible((scene::E_DEBUG_SCENE_TYPE)(Model->isDebugDataVisible()^scene::EDS_SKELETON));
break;
case 440: // View -> Debug Information
if (Model)
Model->setDebugDataVisible((scene::E_DEBUG_SCENE_TYPE)(Model->isDebugDataVisible()^scene::EDS_MESH_WIRE_OVERLAY));
break;
case 450: // View -> Debug Information
if (Model)
Model->setDebugDataVisible((scene::E_DEBUG_SCENE_TYPE)(Model->isDebugDataVisible()^scene::EDS_HALF_TRANSPARENCY));
break;
case 460: // View -> Debug Information
if (Model)
Model->setDebugDataVisible((scene::E_DEBUG_SCENE_TYPE)(Model->isDebugDataVisible()^scene::EDS_BBOX_BUFFERS));
break;
case 499: // View -> Debug Information
if (Model)
Model->setDebugDataVisible(scene::EDS_FULL);
break;
case 500: // Help->About
showAboutText();
break;
case 610: // View -> Material -> Solid
if (Model)
Model->setMaterialType(video::EMT_SOLID);
break;
case 620: // View -> Material -> Transparent
if (Model)
Model->setMaterialType(video::EMT_TRANSPARENT_ADD_COLOR);
break;
case 630: // View -> Material -> Reflection
if (Model)
Model->setMaterialType(video::EMT_SPHERE_MAP);
break;
case 1000:
setActiveCamera ( Camera[0] );
break;
case 1100:
setActiveCamera ( Camera[1] );
break;
case 5001:
printf("Add Sphere\n");
scene::ISceneNode *n1 = Device->getSceneManager()->addSphereSceneNode();
n1->setScale(core::vector3df(2,2,2));
break;
}
break;
}
case EGET_FILE_SELECTED:
{
// load the model file, selected in the file open dialog
IGUIFileOpenDialog* dialog =
(IGUIFileOpenDialog*)event.GUIEvent.Caller;
loadModel(core::stringc(dialog->getFileName()).c_str());
}
case EGET_SCROLL_BAR_CHANGED:
// control skin transparency
if (id == 104)
{
s32 pos = ((IGUIScrollBar*)event.GUIEvent.Caller)->getPos();
for (s32 i=0; i<irr::gui::EGDC_COUNT ; ++i)
{
video::SColor col = env->getSkin()->getColor((EGUI_DEFAULT_COLOR)i);
col.setAlpha(pos);
env->getSkin()->setColor((EGUI_DEFAULT_COLOR)i, col);
}
}
break;
case EGET_COMBO_BOX_CHANGED:
// control anti-aliasing/filtering
if (id == 108)
{
s32 pos = ((IGUIComboBox*)event.GUIEvent.Caller)->getSelected();
switch (pos)
{
case 0:
if (Model)
{
Model->setMaterialFlag(video::EMF_BILINEAR_FILTER, false);
Model->setMaterialFlag(video::EMF_TRILINEAR_FILTER, false);
Model->setMaterialFlag(video::EMF_ANISOTROPIC_FILTER, false);
}
break;
case 1:
if (Model)
{
Model->setMaterialFlag(video::EMF_BILINEAR_FILTER, true);
Model->setMaterialFlag(video::EMF_TRILINEAR_FILTER, false);
}
break;
case 2:
if (Model)
{
Model->setMaterialFlag(video::EMF_BILINEAR_FILTER, false);
Model->setMaterialFlag(video::EMF_TRILINEAR_FILTER, true);
}
break;
case 3:
if (Model)
{
Model->setMaterialFlag(video::EMF_ANISOTROPIC_FILTER, true);
}
break;
case 4:
if (Model)
{
Model->setMaterialFlag(video::EMF_ANISOTROPIC_FILTER, false);
}
break;
}
}
break;
case EGET_BUTTON_CLICKED:
switch(id)
{
case 1101:
{
// set scale
gui::IGUIElement* root = env->getRootGUIElement();
core::vector3df scale;
core::stringc s;
s = root->getElementFromId(901, true)->getText();
scale.X = (f32)atof(s.c_str());
s = root->getElementFromId(902, true)->getText();
scale.Y = (f32)atof(s.c_str());
s = root->getElementFromId(903, true)->getText();
scale.Z = (f32)atof(s.c_str());
if (selNode)
selNode->setScale(scale);
}
break;
case 11010:
{
// set translate
gui::IGUIElement* root = env->getRootGUIElement();
core::vector3df translate;
core::stringc s;
s = root->getElementFromId(904, true)->getText();
translate.X = (f32)atof(s.c_str());
s = root->getElementFromId(905, true)->getText();
translate.Y = (f32)atof(s.c_str());
s = root->getElementFromId(906, true)->getText();
translate.Z = (f32)atof(s.c_str());
if (selNode) {
printf("translating to new position\n");
selNode->setPosition( translate );
}
}
break;
case 11011:
{
// set rotate
gui::IGUIElement* root = env->getRootGUIElement();
core::vector3df rotatn;
core::stringc s;
s = root->getElementFromId(907, true)->getText();
rotatn.X = (f32)atof(s.c_str());
s = root->getElementFromId(909, true)->getText();
rotatn.Y = (f32)atof(s.c_str());
s = root->getElementFromId(909, true)->getText();
rotatn.Z = (f32)atof(s.c_str());
if (selNode) {
printf("rotating to new position\n");
selNode->setRotation( rotatn );
}
}
break;
case 1102:
env->addFileOpenDialog(L"Please select a model file to open");
break;
case 1103:
showAboutText();
break;
case 1104:
createToolBox();
break;
case 1105:
env->addFileOpenDialog(L"Please select your game archive/directory");
break;
}
break;
}
return false;
}
if ( event.EventType == EET_MOUSE_INPUT_EVENT ) {
switch(event.MouseInput.Event)
{
case EMIE_MOUSE_WHEEL:
{
}
break;
case EMIE_RMOUSE_PRESSED_DOWN:
{
printf("EMIE_RMOUSE_PRESSED_DOWN\n");
core::position2di p = core::position2di(event.MouseInput.X,event.MouseInput.Y);
printf("X=%d\n", p.X);
printf("Y=%d\n", p.Y);
scene::ISceneCollisionManager *icm = Device->getSceneManager()->getSceneCollisionManager();
scene::ICameraSceneNode *cam = Device->getSceneManager()->getActiveCamera();
//core::line3d<f32> ray = icm->getRayFromScreenCoordinates(core::position2d<s32>(p.X,p.Y),cam);
selNode = icm->getSceneNodeFromScreenCoordinatesBB(core::position2d<s32>(p.X,p.Y),0,false);
//selNode->setScale(core::vector3df(0.5,0.5,0.5));
core::vector3df ap = selNode->getAbsolutePosition();
gui::IGUIElement* root = Device->getSceneManager()->getGUIEnvironment()->getRootGUIElement();
core::stringw str1(ap.X);
root->getElementFromId(904, true)->setText(str1.c_str());
core::stringw str2(ap.Y);
root->getElementFromId(905, true)->setText(str2.c_str());
core::stringw str3(ap.Z);
root->getElementFromId(906, true)->setText(str3.c_str());
printf("aX=%d\n", ap.X);
printf("aY=%d\n", ap.Y);
printf("aZ=%d\n", ap.Z);
}
break;
case EMIE_LMOUSE_PRESSED_DOWN:
{
}
break;
}
}
return false;
}
};
/*
Most of the hard work is done. We only need to create the Irrlicht Engine device
and all the buttons, menus and toolbars.
We start up the engine as usual, using createDevice(). To make our application
catch events, we set our eventreceiver as parameter. The #ifdef WIN32 preprocessor
commands are not necesarry, but I included them to make the tutorial use DirectX on
Windows and OpenGL on all other platforms like Linux.
As you can see, there is also a unusual call to IrrlichtDevice::setResizeAble().
This makes the render window resizeable, which is quite useful for a mesh viewer.
*/
int main()
{
// ask user for driver
video::E_DRIVER_TYPE driverType = video::EDT_DIRECT3D8;
printf("Please select the driver you want for this example:\n"\
" (a) Direct3D 9.0c\n (b) Direct3D 8.1\n (c) OpenGL 1.5\n"\
" (d) Software Renderer\n (e) Burning's Software Renderer\n"\
" (f) NullDevice\n (otherKey) exit\n\n");
char key;
//std::cin >> key;
key ='a';
switch(key)
{
case 'a': driverType = video::EDT_DIRECT3D9;break;
case 'b': driverType = video::EDT_DIRECT3D8;break;
case 'c': driverType = video::EDT_OPENGL; break;
case 'd': driverType = video::EDT_SOFTWARE; break;
case 'e': driverType = video::EDT_BURNINGSVIDEO;break;
case 'f': driverType = video::EDT_NULL; break;
default: return 1;
}
// create device and exit if creation failed
MyEventReceiver receiver;
Device = createDevice(driverType, core::dimension2d<s32>(1024, 768),
16, false, false, false, &receiver);
if (Device == 0)
return 1; // could not create selected driver.
Device->setResizeAble(true);
Device->setWindowCaption(L"Irrlicht Engine - Loading...");
video::IVideoDriver* driver = Device->getVideoDriver();
IGUIEnvironment* env = Device->getGUIEnvironment();
scene::ISceneManager* smgr = Device->getSceneManager();
smgr->getParameters()->setAttribute(scene::COLLADA_CREATE_SCENE_INSTANCES, true);
driver->setTextureCreationFlag(video::ETCF_ALWAYS_32_BIT, true);
smgr->addLightSceneNode();
smgr->addLightSceneNode(0, core::vector3df(50,-50,100), video::SColorf(1.0f,1.0f,1.0f),20000);
// add our media directory as "search path"
Device->getFileSystem()->addFolderFileArchive ( "../../media/" );
/*
The next step is to read the configuration file. It is stored in the xml
format and looks a little bit like this:
<?xml version="1.0"?>
<config>
<startUpModel file="some filename" />
<messageText caption="Irrlicht Engine Mesh Viewer">
Hello!
</messageText>
</config>
We need the data stored in there to be written into the global variables
StartUpModelFile, MessageText and Caption. This is now done using the
Irrlicht Engine integrated XML parser:
*/
// read configuration from xml file
io::IXMLReader* xml = Device->getFileSystem()->createXMLReader(
"config.xml");
while(xml && xml->read())
{
switch(xml->getNodeType())
{
case io::EXN_TEXT:
// in this xml file, the only text which occurs is the messageText
MessageText = xml->getNodeData();
break;
case io::EXN_ELEMENT:
{
if (core::stringw("startUpModel") == xml->getNodeName())
StartUpModelFile = xml->getAttributeValue(L"file");
else
if (core::stringw("messageText") == xml->getNodeName())
Caption = xml->getAttributeValue(L"caption");
}
break;
}
}
if (xml)
xml->drop(); // don't forget to delete the xml reader
/*
That wasn't difficult. Now we'll set a nicer font and create the
Menu. It is possible to create submenus for every menu item. The call
menu->addItem(L"File", -1, true, true); for example adds a new menu
Item with the name "File" and the id -1. The following parameter says
that the menu item should be enabled, and the last one says, that
there should be a submenu. The submenu can now be accessed with
menu->getSubMenu(0), because the "File" entry is the menu item with
index 0.
*/
// set a nicer font
IGUISkin* skin = env->getSkin();
IGUIFont* font = env->getFont("fonthaettenschweiler.bmp");
if (font)
skin->setFont(font);
// create menu
gui::IGUIContextMenu* menu = env->addMenu();
menu->addItem(L"File", -1, true, true);
menu->addItem(L"View", -1, true, true);
menu->addItem(L"Camera", -1, true, true);
menu->addItem(L"Help", -1, true, true);
menu->addItem(L"Insert", -1, true, true);
gui::IGUIContextMenu* submenu;
submenu = menu->getSubMenu(0);
submenu->addItem(L"Open Model File & Texture...", 100);
submenu->addItem(L"Set Model Archive...", 101);
submenu->addSeparator();
submenu->addItem(L"Quit", 200);
submenu = menu->getSubMenu(1);
submenu->addItem(L"toggle sky box visibility", 300);
submenu->addItem(L"toggle model debug information", -1, true, true);
submenu->addItem(L"model material", -1, true, true );
submenu = submenu->getSubMenu(1);
submenu->addItem(L"Off", 400);
submenu->addItem(L"Bounding Box", 410);
submenu->addItem(L"Normals", 420);
submenu->addItem(L"Skeleton", 430);
submenu->addItem(L"Wire overlay", 440);
submenu->addItem(L"Half-Transparent", 450);
submenu->addItem(L"Buffers bounding boxes", 460);
submenu->addItem(L"All", 499);
submenu = menu->getSubMenu(1)->getSubMenu(2);
submenu->addItem(L"Solid", 610);
submenu->addItem(L"Transparent", 620);
submenu->addItem(L"Reflection", 630);
submenu = menu->getSubMenu(2);
submenu->addItem(L"Maya Style", 1000);
submenu->addItem(L"First Person", 1100);
submenu = menu->getSubMenu(3);
submenu->addItem(L"About", 500);
submenu = menu->getSubMenu(4);
submenu->addItem(L"Sphere", 5001);
/*
Below the toolbar, we want a toolbar, onto which we can place
colored buttons and important looking stuff like a senseless
combobox.
*/
// create toolbar
gui::IGUIToolBar* bar = env->addToolBar();
video::ITexture* image = driver->getTexture("open.png");
bar->addButton(1102, 0, L"Open a model",image, 0, false, true);
image = driver->getTexture("tools.png");
bar->addButton(1104, 0, L"Open Toolset",image, 0, false, true);
image = driver->getTexture("zip.png");
bar->addButton(1105, 0, L"Set Model Archive",image, 0, false, true);
image = driver->getTexture("help.png");
bar->addButton(1103, 0, L"Open Help", image, 0, false, true);
// create a combobox with some senseless texts
gui::IGUIComboBox* box = env->addComboBox(core::rect<s32>(250,4,350,23), bar, 108);
box->addItem(L"No filtering");
box->addItem(L"Bilinear");
box->addItem(L"Trilinear");
box->addItem(L"Anisotropic");
box->addItem(L"Isotropic");
/*
To make the editor look a little bit better, we disable transparent
gui elements, and add a Irrlicht Engine logo. In addition, a text
showing the current frame per second value is created and
the window caption is changed.
*/
// disable alpha
for (s32 i=0; i<gui::EGDC_COUNT ; ++i)
{
video::SColor col = env->getSkin()->getColor((gui::EGUI_DEFAULT_COLOR)i);
col.setAlpha(255);
env->getSkin()->setColor((gui::EGUI_DEFAULT_COLOR)i, col);
}
// add a tabcontrol
createToolBox();
// create fps text
IGUIStaticText* fpstext = env->addStaticText(L"", core::rect<s32>(400,4,570,23), true, false, bar);
// set window caption
Caption += " - [";
Caption += driver->getName();
Caption += "]";
Device->setWindowCaption(Caption.c_str());
/*
That's nearly the whole application. We simply show the about
message box at start up, and load the first model. To make everything
look better, a skybox is created and a user controled camera,
to make the application a little bit more interactive. Finally,
everything is drawed in a standard drawing loop.
*/
// show about message box and load default model
//showAboutText();
loadModel(StartUpModelFile.c_str());
// add skybox
SkyBox = smgr->addSkyBoxSceneNode(
driver->getTexture("irrlicht2_up.jpg"),
driver->getTexture("irrlicht2_dn.jpg"),
driver->getTexture("irrlicht2_lf.jpg"),
driver->getTexture("irrlicht2_rt.jpg"),
driver->getTexture("irrlicht2_ft.jpg"),
driver->getTexture("irrlicht2_bk.jpg"));
// add billboard
scene::IBillboardSceneNode * bill = smgr->addBillboardSceneNode();
bill->setMaterialType(video::EMT_TRANSPARENT_ADD_COLOR );
bill->setMaterialTexture(0, driver->getTexture("../../media/particle.bmp"));
bill->setMaterialFlag(video::EMF_LIGHTING, false);
bill->setMaterialFlag(video::EMF_ZBUFFER, false);
bill->setSize(core::dimension2d<f32>(4.0f, 4.0f));
video::SMaterial material;
material.setTexture(0, driver->getTexture("../../media/faerie2.bmp"));
material.Lighting = true;
// add a camera scene node
Camera[0] = smgr->addCameraSceneNodeMaya();
Camera[1] = smgr->addCameraSceneNodeFPS();
setActiveCamera ( Camera[0] );
scene::ITriangleSelector* selector = 0;
Camera[0]->setTriangleSelector(selector);
Camera[1]->setTriangleSelector(selector);
// load the irrlicht engine logo
IGUIImage *img =
env->addImage(driver->getTexture("irrlichtlogo2.png"),
core::position2d<s32>(10, driver->getScreenSize().Height - 128));
// lock the logo's edges to the bottom left corner of the screen
img->setAlignment(EGUIA_UPPERLEFT, EGUIA_UPPERLEFT, EGUIA_LOWERRIGHT, EGUIA_LOWERRIGHT);
// draw everything
while(Device->run() && driver)
{
if (Device->isWindowActive())
{
driver->beginScene(true, true, video::SColor(150,50,50,50));
smgr->drawAll();
env->drawAll();
if ( selNode > 0 ) {
core::vector3df p = selNode->getAbsolutePosition();
//printf("X=%d\n", p.X);
//printf("Y=%d\n", p.Y);
//printf("Z=%d\n", p.Z);
//core::matrix4 mtx;
//mtx.setTranslation(selNode->getAbsolutePosition());
//mtx.setRotationDegrees(selNode->getRotation());
//mtx.setScale(selNode->getScale());
//driver->setTransform(video::ETS_WORLD, mtx);
driver->setTransform(video::ETS_WORLD, core::matrix4());
driver->setMaterial(material);
bill->setPosition(p);
core::vector3df p1(p.X+40, p.Y, p.Z);
core::vector3df p2(p.X, p.Y+40, p.Z);
core::vector3df p3(p.X, p.Y, p.Z+40);
driver->draw3DLine(p,p1,video::SColor(255,0,0,255));
driver->draw3DLine(p,p2,video::SColor(255,0,255,0));
driver->draw3DLine(p,p3,video::SColor(255,255,0,0));
/*
core::stringc nm1(L"handleX");
core::stringc nm2(L"handleY");
core::stringc nm3(L"handleZ");
scene::IAnimatedMesh *h1 = Device->getSceneManager()->addArrowMesh(nm1.c_str(), video::SColor(255,0,0,255), video::SColor(255,0,0,255), 4, 8, 25, 15, 2, 2);
scene::IAnimatedMeshSceneNode* am1 = Device->getSceneManager()->addAnimatedMeshSceneNode(h1,0,-1,p,core::vector3df(0,0,-90));
am1->setPosition(p);
scene::IAnimatedMesh *h2 = Device->getSceneManager()->addArrowMesh(nm2.c_str(), video::SColor(255,255,0,0), video::SColor(255,255,0,0), 4, 8, 25, 15, 2, 2);
scene::IAnimatedMeshSceneNode* am2 = Device->getSceneManager()->addAnimatedMeshSceneNode(h2,0,-1,p,core::vector3df(90,0,0));
scene::IAnimatedMesh *h3 = Device->getSceneManager()->addArrowMesh(nm3.c_str(), video::SColor(255,0,255,0), video::SColor(255,0,255,0), 4, 8, 25, 15, 2, 2);
scene::IAnimatedMeshSceneNode* am3 = Device->getSceneManager()->addAnimatedMeshSceneNode(h3,0,-1,p,core::vector3df(0,0,0));
*/
core::matrix4 mtx2;
mtx2.setTranslation(selNode->getAbsolutePosition());
mtx2.setRotationDegrees(selNode->getRotation());
mtx2.setScale(selNode->getScale());
driver->setTransform(video::ETS_WORLD, mtx2);
driver->draw3DBox(selNode->getBoundingBox(), SColor(255, 255, 255, 255));
}
driver->endScene();
core::stringw str(L"FPS: ");
str.append(core::stringw(driver->getFPS()));
str += L" Tris: ";
str.append(core::stringw(driver->getPrimitiveCountDrawn()));
fpstext->setText(str.c_str());
}
else
Device->yield();
}
Device->drop();
return 0;
}
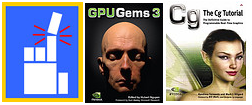
If you're actually going to make an open source scene editor, I have two suggestions..
1) serialize the node's attributes to an attribute editor element (see the gui editor)
2) forum posts aren't the best place for the code, especially with one post for each revision
if it's going to be a simple scene editor as a code snippet, do it as one post in the code snippets forum
1) serialize the node's attributes to an attribute editor element (see the gui editor)
2) forum posts aren't the best place for the code, especially with one post for each revision
