Area Damage for Rockets, Grenades etc.
-
- Posts: 58
- Joined: Tue Apr 10, 2007 7:49 pm
- Location: Karlsruhe
Area Damage for Rockets, Grenades etc.
I would like an opponent to take damage when i fired a rocket on him, that did not actually
hit him, but hit the ground close to him.
Thus i would like to have a list of all ISceneNodes in some sphere. I would then calculate for
all these SceneNodes there distance to the impact and apply damage accordingly.
Now, how do i get such a list of SceneNodes in some sphere?
I think the buildin CollisionSystem does not work for me in this case.
Any ideas?
hit him, but hit the ground close to him.
Thus i would like to have a list of all ISceneNodes in some sphere. I would then calculate for
all these SceneNodes there distance to the impact and apply damage accordingly.
Now, how do i get such a list of SceneNodes in some sphere?
I think the buildin CollisionSystem does not work for me in this case.
Any ideas?
-
- Posts: 25
- Joined: Sat Jun 07, 2008 8:55 pm
well a "sphere" could be thought as a distance to target really.
if "this node" is "this far" from "impact position" then apply "this damage"
You could then depending on the distance between positions of the node and impact spot set a fall off damage from the distance value.
edit: so to get all nodes within a certain spherical distance your just looking for the radius length between nodes in relation to the impact point of the rocket.
if "this node" is "this far" from "impact position" then apply "this damage"
You could then depending on the distance between positions of the node and impact spot set a fall off damage from the distance value.
edit: so to get all nodes within a certain spherical distance your just looking for the radius length between nodes in relation to the impact point of the rocket.
--
Juicy, hot, and full of butter!
Juicy, hot, and full of butter!
-
- Posts: 58
- Joined: Tue Apr 10, 2007 7:49 pm
- Location: Karlsruhe
Comparing calculating the distance between two nodes and comparing this distance with another value doesn't take ages.
If this is really too slow you could use a bsp tree or something similar. So you only have to check nodes next to the position.
If this is really too slow you could use a bsp tree or something similar. So you only have to check nodes next to the position.
Software documentation is like sex. If it's good you want more. If it's bad it's better than nothing.
Yeah if you make some sort of quick sort algorithm, you can quickly select the nodes that are affected, and them, manage the damage, etc...
OR, you can make each enemy objetc to check the colision at every loop....
Anywaysm, you can always make siple apps to make tests and then choose the best way....
OR, you can make each enemy objetc to check the colision at every loop....
Anywaysm, you can always make siple apps to make tests and then choose the best way....
-
- Posts: 914
- Joined: Fri Aug 03, 2007 12:43 pm
- Location: South Africa
- Contact:
Normally, a rocket has an on collision event. The players/enemies will have a bounding box, and so can the rocket itself.
So all you need is rocket bounding box and player bounding box. On rocket collision : (inside gamerocket.cpp for example)
if there are 16 players (which is a LOT) its still not a lot of checks on a bounding box.
if rocket.boundingbox contains/intersects with (any of the player boxes), calculate the distance and apply force.
getDistanceAndDirection is obvious too,
dir = (rocketPos - PlayerPos) is the "direction" of the force, and
dir.normalise will give you a value between 0 and 1 for the force "amount". You can then lerp the values between max rocket damage, and that percent of damage.
So all you need is rocket bounding box and player bounding box. On rocket collision : (inside gamerocket.cpp for example)
if there are 16 players (which is a LOT) its still not a lot of checks on a bounding box.
if rocket.boundingbox contains/intersects with (any of the player boxes), calculate the distance and apply force.
Code: Select all
in void onRocketCollision()
{
for each player
if rocket.boundingbox intersects/contains players[i].boundingbox
damage = getDistanceAndDirectionFromPlayer (players[i]);
applyForceToPlayer(damage);
}
dir = (rocketPos - PlayerPos) is the "direction" of the force, and
dir.normalise will give you a value between 0 and 1 for the force "amount". You can then lerp the values between max rocket damage, and that percent of damage.
-
- Posts: 58
- Joined: Tue Apr 10, 2007 7:49 pm
- Location: Karlsruhe
why are u checking for all scenenodes? is really every scenenode going to be affectd by the rocket?
We're programmers. Programmers are, in their hearts, architects, and the first thing they want to do when they get to a site is to bulldoze the place flat and build something grand. We're not excited by renovation:tinkering,improving,planting flower beds.
-
- Posts: 58
- Joined: Tue Apr 10, 2007 7:49 pm
- Location: Karlsruhe
Well, i will check for those, that might be affected.
My question was, if i can exclude SceneNodes from distance testing that are way out of
range somehow, and if irrlicht offers some facilities to do so efficiently.
Currently, there aren't so many SceneNodes in my game, so testing them all is feasible.
In a later state however, the number of SceneNodes may forbid such a trivial approach.
I'm planning to check out physic engines later, maybe i'll find a better solution then.
My question was, if i can exclude SceneNodes from distance testing that are way out of
range somehow, and if irrlicht offers some facilities to do so efficiently.
Currently, there aren't so many SceneNodes in my game, so testing them all is feasible.
In a later state however, the number of SceneNodes may forbid such a trivial approach.
I'm planning to check out physic engines later, maybe i'll find a better solution then.
well.. you could test their all their x, y, z distances to make sure they are under a certain distance, and if all of them are then do the sqrt(x*x + y*y + z*z) so that you quickly check the masses but slowly check the probables.
a compare function like if(x<50) is like 3 or 4 cpu cycles, but a x*x is like 40.
a compare function like if(x<50) is like 3 or 4 cpu cycles, but a x*x is like 40.
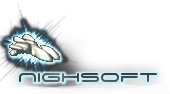
CvIrrCamController - 3D head tracking lib to create window effect with webcam
IrrAR - Attach Irrlicht nodes to real life markers
http://www.nighsoft.com/
ok something really weird happend i just wrote a octTree class to do the quick bounding box lookup search.
I tried with 10000 scenenodes....took 0 ms to find the nodes in range.
then i did a simple list traversal and checked all scenenodes. this also took 0 ms...mesured with clock().
I tried with 10000 scenenodes....took 0 ms to find the nodes in range.
then i did a simple list traversal and checked all scenenodes. this also took 0 ms...mesured with clock().
We're programmers. Programmers are, in their hearts, architects, and the first thing they want to do when they get to a site is to bulldoze the place flat and build something grand. We're not excited by renovation:tinkering,improving,planting flower beds.
You are probably not performing any "changes" to anything outside the loop, therefore it would get optimized out.
E.g:
vs
Here is a great resource for reference: http://www.agner.org/optimize/optimizing_cpp.pdf
E.g:
Code: Select all
for
{
var2 = 5;
}
Code: Select all
var = 0;
for
{
var2 = 5;
var += var2;
}
Sadly this is only true for very old processors. These days the cost of a branch is much higher.a compare function like if(x<50) is like 3 or 4 cpu cycles, but a x*x is like 40.
Here is a great resource for reference: http://www.agner.org/optimize/optimizing_cpp.pdf
ShadowMapping for Irrlicht!: Get it here
Need help? Come on the IRC!: #irrlicht on irc://irc.freenode.net
Need help? Come on the IRC!: #irrlicht on irc://irc.freenode.net