hi sirs,
I think there is a slight bug on device->getFileSystem()->addZipFileArchive(); but it isn't really that critical. In fact, the condition is rare and can easily be avoided with a simple workaround. I just reported this for documentation purposes only. ^_^
The bug can be described as follows:
If you have 2 identical filenames on 2 different directories, one of them is always loaded even if you specify the directory of the second. So far, I've been able to recreate the conditions always and on both windows and linux.
The code below describes it better, also found below are the links to my test case which contains the test source code and the test zip file.
The zip file contains 3 files and 1 folder in this format:
Code: Select all
vfs.zip
- vfs
- dir1
- 1.jpg
- 1.jpg
- 2.jpg
dir1/1.jpg contains image of 1s
1.jpg contains images of 2s despite the filename
2.jpg contains images of 2s
in other words, both 1.jpg and 2.jpg outside of dir1 contains image of 2s,
while the image at dir1/1.jpg contains image of 1s.
Viewing the source code, I created 3 cubes
cube 1 uses vfs/1.jpg as texture
cube 2 uses vfs/dir1/1.jpg as texture
cube 3 uses vfs/2.jpg as texture
The expected output should be cubes with textures 2s, 1s and 2s respectively. However, upon running, the output is different.
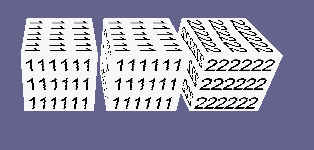
The workaround is simple, don't use exactly the same filename even across different folders.
Following is the link to the test case.
source code and assets
It contains the zip file, the source codes, and binary for windows 32bit and linux 64bit.
If i'm done with my projects, i'll probably try looking at the source code and see if i can manage to create a patch for it. For now, all I can do is report for documentation. ^_^
Code: Select all
#include <irrlicht.h>
using namespace irr;
using namespace core;
using namespace scene;
using namespace video;
using namespace io;
using namespace gui;
#ifdef _IRR_WINDOWS_
#pragma comment(lib, "Irrlicht.lib")
#pragma comment(linker, "/subsystem:windows /ENTRY:mainCRTStartup")
#endif
int main()
{
IrrlichtDevice *device =
createDevice( video::EDT_SOFTWARE, dimension2d<u32>(640, 480), 16,
false, false, false, 0);
if (!device)
return 1;
device->setWindowCaption(L"Irrlicht Engine Test");
device->getFileSystem()->addZipFileArchive("vfs.zip");
IVideoDriver* driver = device->getVideoDriver();
ISceneManager* smgr = device->getSceneManager();
// 1st cube
ISceneNode* cube1 = smgr->addCubeSceneNode(10.0f);
if (!cube1)
{
device->drop();
return 1;
}
cube1->setMaterialTexture( 0, driver->getTexture("vfs/1.jpg") ); // an image with 2s
cube1->setMaterialFlag(EMF_LIGHTING, false);
// 2nd cube
ISceneNode* cube2 = smgr->addCubeSceneNode(10.0f);
if (!cube2)
{
device->drop();
return 1;
}
cube2->setPosition(vector3df(12,0,0));
cube2->setMaterialTexture( 0, driver->getTexture("vfs/dir1/1.jpg") ); // an image with 1s
cube2->setMaterialFlag(EMF_LIGHTING, false);
// 3rd cube
ISceneNode* cube3 = smgr->addCubeSceneNode(10.0f);
if (!cube3)
{
device->drop();
return 1;
}
cube3->setPosition(vector3df(24,0,0));
cube3->setMaterialTexture( 0, driver->getTexture("vfs/2.jpg") ); // an image with 2s
cube3->setMaterialFlag(EMF_LIGHTING, false);
smgr->addCameraSceneNode(0, vector3df(0,30,-40), vector3df(0,5,0));
while(device->run())
{
driver->beginScene(true, true, SColor(255,100,101,140));
smgr->drawAll();
driver->endScene();
}
device->drop();
return 0;
}