The top left pixel from the rectangle is missing
Using latest revision from Irrlicht trunk.
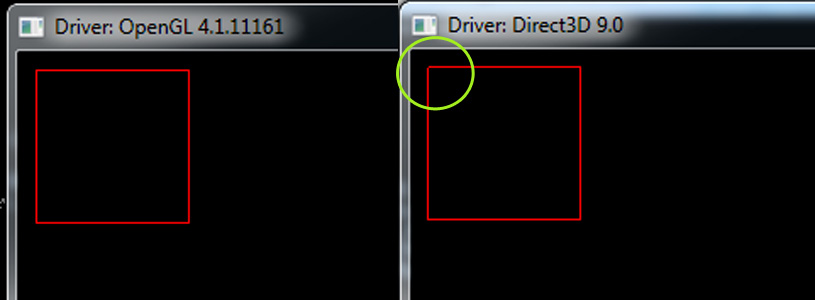
Testcase:
Code: Select all
#include <irrlicht.h>
#include "driverChoice.h"
using namespace irr;
int main() {
// ask user for driver
video::E_DRIVER_TYPE driverType = driverChoiceConsole();
if (driverType == video::EDT_COUNT)
return 1;
// create device with full flexibility over creation parameters
// you can add more parameters if desired, check irr::SIrrlichtCreationParameters
irr::SIrrlichtCreationParameters params;
params.DriverType = driverType;
params.WindowSize = core::dimension2d<u32>(1024, 768);
params.Bits = 32;
IrrlichtDevice* device = createDeviceEx(params);
if (device == 0)
return 1; // could not create selected driver.
video::IVideoDriver* driver = device->getVideoDriver();
scene::ISceneManager* smgr = device->getSceneManager();
gui::IGUIEnvironment* env = device->getGUIEnvironment();
// Set window title
core::stringw str = L"Driver: ";
str += driver->getName();
device->setWindowCaption(str.c_str());
while (device->run()) {
if (device->isWindowActive()) {
driver->beginScene(true, true, 0);
smgr->drawAll();
env->drawAll();
// Draw rectangle
irr::core::recti rect = irr::core::recti(10,10, 100,100);
driver->draw2DRectangleOutline(rect, video::SColor(255,255,0,0));
// test line
driver->draw2DLine(core::vector2di(10,8), core::vector2di(100,8), video::SColor(255,255,0,0));
driver->endScene();
}
}
device->drop();
return 0;
}
draw2Dline() seems to be the problem
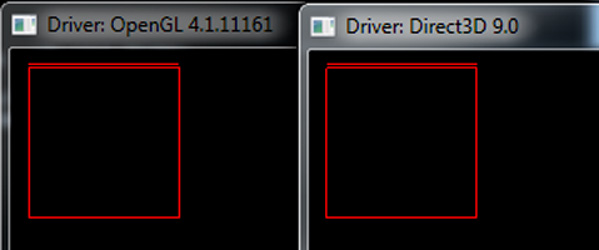