First row of text are drawn to a RTT, second normal font drawing.
I used 4 font supplied in irrlicht.
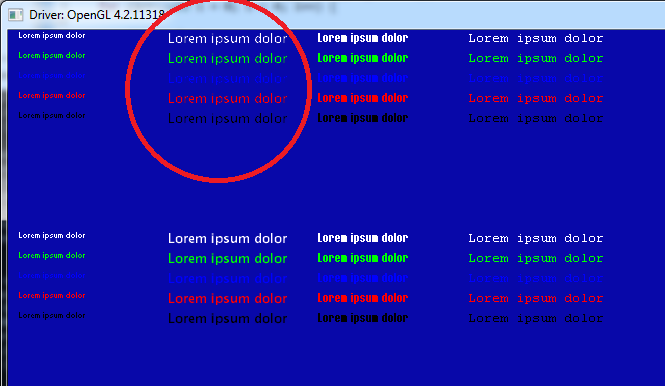
Simple testcase:
Code: Select all
#include <irrlicht.h>
#include "driverChoice.h"
using namespace irr;
// Draw fonts function
void drawFonts(gui::IGUIEnvironment* env, irr::u32 y) {
gui::IGUIFont* font = env->getSkin()->getFont();
for (irr::u32 i = 0; i < 4; i++) {
// Get font
if (i == 1) {
font = env->getFont("fontlucida.png"); // Has alpha
} else if (i == 2) {
font = env->getFont("fonthaettenschweiler.bmp");
} else if (i == 3) {
font = env->getFont("fontcourier.bmp");
}
// Draw text
font->draw(L"Lorem ipsum dolor", core::recti(10 + (150 * i), y, 100 + (150 * i), y + 20), video::SColor(255, 255, 255, 255));
font->draw(L"Lorem ipsum dolor", core::recti(10 + (150 * i), y + 20, 100 + (150 * i), y + 40), video::SColor(255, 0, 255, 0));
font->draw(L"Lorem ipsum dolor", core::recti(10 + (150 * i), y + 40, 100 + (150 * i), y + 60), video::SColor(255, 0, 0, 255));
font->draw(L"Lorem ipsum dolor", core::recti(10 + (150 * i), y + 60, 100 + (150 * i), y + 80), video::SColor(255, 255, 0, 0));
font->draw(L"Lorem ipsum dolor", core::recti(10 + (150 * i), y + 80, 100 + (150 * i), y + 100), video::SColor(255, 0, 0, 0));
}
}
int main() {
// ask user for driver
video::E_DRIVER_TYPE driverType = driverChoiceConsole();
if (driverType == video::EDT_COUNT)
return 1;
// create device with full flexibility over creation parameters
// you can add more parameters if desired, check irr::SIrrlichtCreationParameters
irr::SIrrlichtCreationParameters params;
params.DriverType = driverType;
params.WindowSize = core::dimension2d<u32>(1024, 768);
params.Bits = 32;
IrrlichtDevice* device = createDeviceEx(params);
if (device == 0)
return 1; // could not create selected driver.
video::IVideoDriver* driver = device->getVideoDriver();
scene::ISceneManager* smgr = device->getSceneManager();
gui::IGUIEnvironment* env = device->getGUIEnvironment();
if (!driver->queryFeature(video::EVDF_RENDER_TO_TARGET)) {
printf("EVDF_RENDER_TO_TARGET not supported!!\n");
}
// Create RTT
core::dimension2du dimension = core::dimension2du(800, 400);
video::ITexture* texture = driver->addRenderTargetTexture(dimension, "RTT", video::ECF_A8R8G8B8);
// Set window title
core::stringw str = L"Driver: ";
str += driver->getName();
device->setWindowCaption(str.c_str());
const video::SColor color = video::SColor(255, 255, 255, 255);
while (device->run()) {
if (device->isWindowActive()) {
if (texture) {
driver->setRenderTarget(texture, true, true, video::SColor(0, 0, 0, 0));
driver->draw2DRectangle(video::SColor(100, 0, 0, 0), core::recti(0, 0, 800, 400));
// Draw fonts
drawFonts(env, 0);
driver->setRenderTarget(0, false, false, 0);
}
driver->beginScene(true, true, video::SColor(255, 10, 10, 200));
smgr->drawAll();
env->drawAll();
// Draw RTT
driver->draw2DImage(texture, core::vector2di(0, 0), core::recti(0, 0, texture->getOriginalSize().Width, texture->getOriginalSize().Height), 0, color, true);
// Draw fonts normal
drawFonts(env, 200);
driver->endScene();
}
}
device->drop();
return 0;
}
Both DirectX and OpenGL have this problem.