I'm using Bullet Physics, and have implemented a custom MotionState that updates the Irrlicht nodes position and rotation to that of the rigid body.
My first iteration of the function simply created new Irr nodes for each sub-cube. Obviously that sucked up a lot of fps and was not ideal.
So my question is two parts,
1. What would be the best way to optimize the rendering of many cubes that are each animated by their own Bullet rigid body?
I'm working on a possible solution; that is to add each sub-cube manually to the mesh buffers. One per buffer. Then when the rigid body calls its MotionState, update the vertices of that buffer manually.
I've got a working MotionState that updates the position of the vertices, but
2. Is there a simple way to apply transforms to a MeshBuffer?
Currently I am updating the positions manually, I wonder if there is a better way to apply Bullet's btTransform to the mesh buffer?
Relevant code:
Code: Select all
class myMotionState : public btDefaultMotionState
{
myCube* cube;
...
void setWorldTransform (const btTransform& centerOfMassWorldTrans)
{
if (cube->isFractured)
{
SMeshBuffer* buf = (SMeshBuffer*) m_cube->node->getMesh()->getMeshBuffer(0);
btVector3 euler;
k.QuaternionToEuler (centerOfMassWorldTrans.getRotation(), euler);
vector3df rot = vector3df (euler[0], euler[1], euler[2]);
matrix4 m;
m.setRotationDegrees (rot);
for (u16 i=0; i<buf->Vertices.size(); i++)
{
vector3df rotPos = m_cube->origVertices[i].Pos;
m.rotateVect (rotPos);
buf->Vertices[i].Pos = rotPos + newPos;
}
buf->recalculateBoundingBox();
}
}
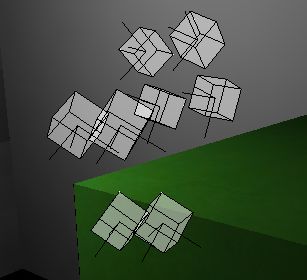