Code: Select all
#include <irrlicht.h>
using namespace irr;
using namespace std;
using namespace core;
using namespace scene;
using namespace video;
using namespace io;
using namespace gui;
int main()
{
IrrlichtDevice *device = createDevice(EDT_OPENGL, dimension2d<s32>(800, 600), 16,false, false, false, 0);
device->setWindowCaption(L"Test Particle System");
IVideoDriver* driver = device->getVideoDriver();
ISceneManager* smgr = device->getSceneManager();
IGUIEnvironment* guienv = device->getGUIEnvironment();
/* Get the mesh of a rocket from "media" directory */
IAnimatedMesh *meshMissile = smgr->getMesh("media/rocket.3ds");
/* Create an animatedMeshSceneNode by default in 0,0,0 with the mesh of the rocket */
IAnimatedMeshSceneNode *nodeMissile = smgr->addAnimatedMeshSceneNode( meshMissile );
nodeMissile->setMaterialFlag(EMF_LIGHTING, false);
/*
Now create a particle system with the idea of being the rocket thruster.
Since usually in a missile both thruster and rocket itself move all togheter
I thought it was a good idea having particle system thruster as childNode of rocket.
Rocket is directed inside the screen and fire of thruster should be directed out from his back.
If we don't use any offset between father and child nodes, particle system will be inside rocket and maybe not visible
so child node particle system should be positioned a little back relatively to his father. Anyway NOTHING is telling
ANY NODE to MOVE from its position ok?
*/
IParticleSystemSceneNode *nodoFireMissile = smgr->addParticleSystemSceneNode(false, nodeMissile, -1,vector3df(0.0,0.0,-1.0));
nodoFireMissile->setParticleSize(dimension2d<f32>(2.0,2.0));
IParticleEmitter *emitterFireMissile = nodoFireMissile->createPointEmitter(
vector3df(0.000f,0.00f,-0.009f),
70,85, SColor(0, 255, 255, 255), SColor(0, 255, 255, 0), 200, 700, 2);
nodoFireMissile->setEmitter(emitterFireMissile);
emitterFireMissile->drop();
if (nodoFireMissile) {
nodoFireMissile->setMaterialFlag(EMF_LIGHTING, false);
nodoFireMissile->setMaterialTexture(0, driver->getTexture("media/ParticleFire.tga"));
nodoFireMissile->setMaterialType(EMT_TRANSPARENT_ADD_COLOR);
}
smgr->addCameraSceneNode(0, vector3df(0,20,-25), vector3df(0,5,0));
while(device->run())
{
driver->beginScene(true, true, SColor(0,200,200,200));
smgr->drawAll();
guienv->drawAll();
driver->endScene();
}
device->drop();
return 0;
}
Though the strange thing is that if I use an offset vecto3df in addParticleSystemSceneNode method, the particle system unexpectly moves away from his father and keep moving far and far. If I don't use offset in addParticleSystemSceneNode method, the particle system doesn't move yet it is exactly at the same coords as rocket so it apper inside rocket. If I try to manually position child I get the same moving away effect. Another strange thing is that the speed of how fast the child node moves away is proportional to vector3df of direction in createPointEmitter method, that is if I use a direction vector3df(0.0f,0.0f,0.0f) while creating emitter, particle system doesn't move from his father but this way I loose the "fire effect".So this is what happens:
Program starts:
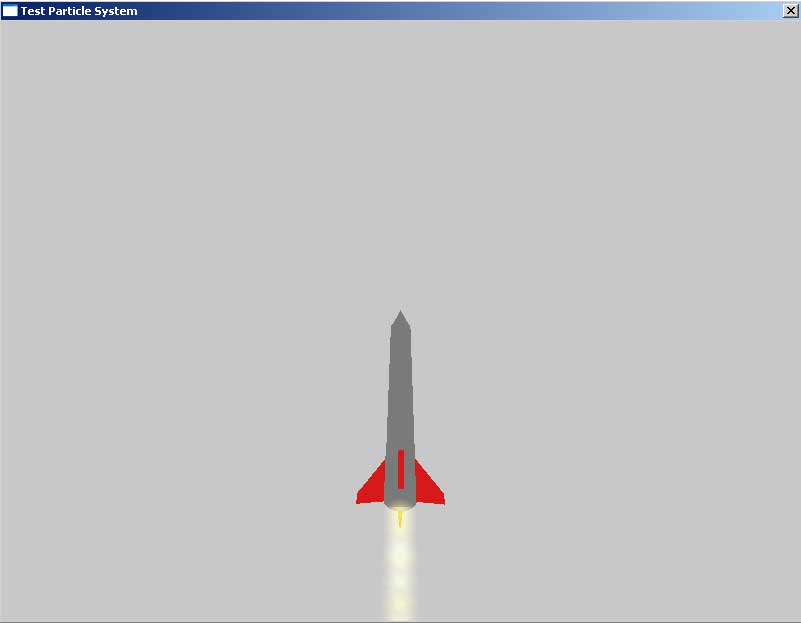
After one second or two:
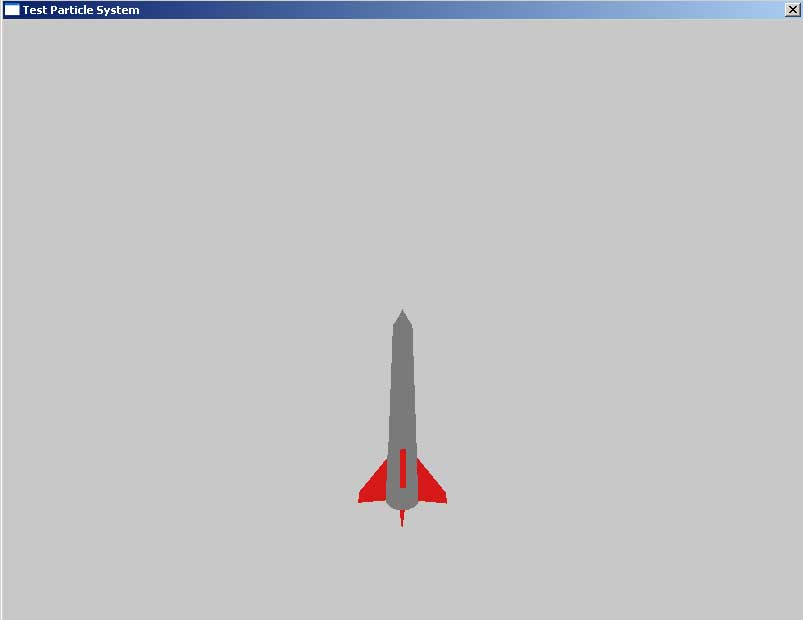
Medias for this testing prgram can be downloaded here:
http://www.manicomio.org/levante/media.zip
Please help me, I think Particle system should behave differently

Marco
[/img][/url][/code]