
I know this has probably been done a million times. But I like to share, okay? I'm learning vc++ and winforms programming. So, hopefully someone would find the code and tool useful. I found most of my answers by searching the irrlicht forum. You can find some excellent info on this forum.
Anyway, here's what it can currently do (usual stuff):
+ fullscreen option on/off
+ stencil buffer option on/off
+ vsync option on/off
+ display driver
+ screen resolution height
+ screen resolution weight
+ screen resolution bits
+ read in values from xml file
+ minimal error checking
I'm currently working on getting it to write to xml. Also, I'm going to add in more error checking code after I've completed the code to allow it to write to xml. I'm also planning a x64 and a .NET 3.0 version (vista only). But let's not get ahead of ourself, eh?

Here're some screen captures of the program (i'm running vista that's why the window looks so funky; also ignore the background -- man, that would be nice to have a grass node looking like that, eh? *lug nudges bitplane*

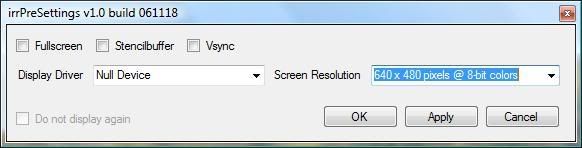
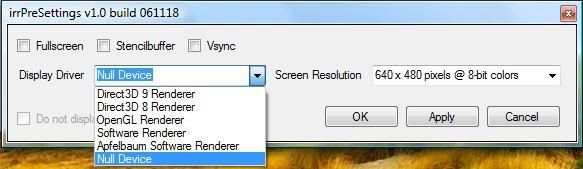
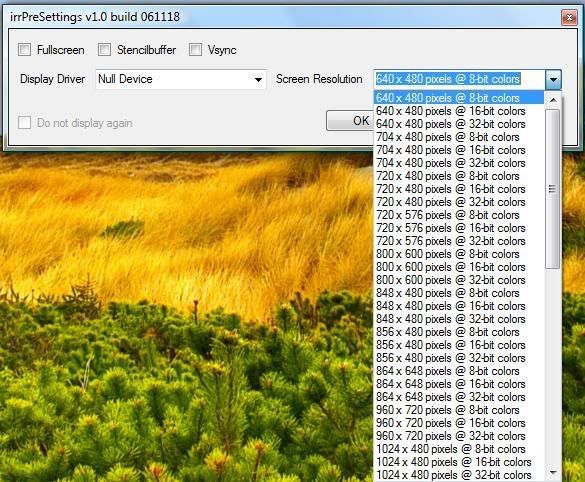
Here's the xml file that the program uses:
Code: Select all
<!-- Pre Setting Configuration File -->
<Config>
<!-- Misc Options -->
<Fullscreen value = "False" />
<Stencilbuffer value = "False" />
<Vsync value = "False" />
<!-- Display Options -->
<DisplayDriver type = "Null Device" />
<ScreenResHeight value = "640" />
<ScreenResWidth value = "480" />
<ScreenResBits value = "8" />
</Config>
I used the "/clr" switch instead of the "/clr:safe" or "/clr:pure" switch in order to take advantage of the non-.NET version of irrlicht. I can't seem to find the operation to get the mode list in the .NET version of irrlicht.
This is pretty much the main guts of the code since everything right now is done when the form first loads:
Code: Select all
private: System::Void Form1_Load(System::Object^ sender, System::EventArgs^ e)
{
// instantiate a dummy irrlicht device
IrrlichtDevice * device = createDevice(
video::EDT_NULL,
core::dimension2d<s32>(0,0),
false,
false);
// query the device to obtain the available video mode
IVideoModeList * availableVideoModeList = device->getVideoModeList();
// define array to hold a formatted listing of the availabe modes
wchar_t formated_listing[64] = L" ";
// go through all available video mode for graphic adapter
for(int i = 0; i < availableVideoModeList->getVideoModeCount(); ++i)
{
// format available modes into desired listing
swprintf(
formated_listing,
64,
L"%i x %i pixels @ %i-bit colors",
availableVideoModeList->getVideoModeResolution(i).Width,
availableVideoModeList->getVideoModeResolution(i).Height,
availableVideoModeList->getVideoModeDepth(i));
// convert wchar_t to string object
System::String^ mode_listing = gcnew System::String(formated_listing);
// add formatted mode to combo list box
cmbScreenResolution->Items->Add(mode_listing);
}
// load in values from xml file if one already exists
io::IXMLReader * xml_file = device->getFileSystem()->createXMLReader("pre_settings.xml");
// check if opened successfully or not (0)
if(xml_file == 0)
{
// show popup saying file could not be found
MessageBox::Show(
"Missing xml setting config file! Falling back to default settings",
"The file \"pre_settings.xml\" cannot be found.",
MessageBoxButtons::OK,
MessageBoxIcon::Exclamation);
}
// define arrays to hold a xml screen resolution values
wchar_t formatted_height[64] = L" ";
wchar_t formatted_width[64] = L" ";
wchar_t formatted_bits[64] = L" ";
// parse through the xml file
while(xml_file && xml_file->read())
{
// current XML node
switch(xml_file->getNodeType())
{
// xml element
case io::EXN_ELEMENT:
// go through all the xml element in file and set
// the window controls as appropriate
if(!std::wcscmp(L"Fullscreen",xml_file->getNodeName()))
{
// default to safe value
cbFullScreen->Checked = false;
// value is true
if(!std::wcscmp(L"True",xml_file->getAttributeValue(L"value")))
{
// set control to checked
cbFullScreen->Checked = true;
}
}
else if(!std::wcscmp(L"Stencilbuffer",xml_file->getNodeName()))
{
// default to safe value
cbStencilBuffer->Checked = false;
// value is true
if(!std::wcscmp(L"True",xml_file->getAttributeValueSafe(L"value")))
{
// set control to checked
cbStencilBuffer->Checked = true;
}
}
else if(!std::wcscmp(L"Vsync",xml_file->getNodeName()))
{
// default to safe value
cbVsync->Checked = false;
// value is true
if(!std::wcscmp(L"True",xml_file->getAttributeValueSafe(L"value")))
{
// set control to checked
cbVsync->Checked = true;
}
}
else if(!std::wcscmp(L"DisplayDriver",xml_file->getNodeName()))
{
// look for "Null Device" index value in the list of drivers
int index = cmbDisplayDriver->FindString("Null Device");
// set the combobox to show the found index to use as default driver
cmbDisplayDriver->SelectedIndex = index;
// read driver string from xml and set the correct index in combobox
if(!std::wcscmp(L"EDT_SOFTWARE",xml_file->getAttributeValueSafe(L"type")))
{
int index = cmbDisplayDriver->FindString("Software Renderer");
cmbDisplayDriver->SelectedIndex = index;
}
else
if(!std::wcscmp(L"EDT_SOFTWARE2",xml_file->getAttributeValueSafe(L"type")))
{
int index = cmbDisplayDriver->FindString("Apfelbaum Software Renderer");
cmbDisplayDriver->SelectedIndex = index;
}
else
if(!std::wcscmp(L"EDT_DIRECT3D8",xml_file->getAttributeValueSafe(L"type")))
{
int index = cmbDisplayDriver->FindString("Direct3D 8 Renderer");
cmbDisplayDriver->SelectedIndex = index;
}
else
if(!std::wcscmp(L"EDT_DIRECT3D9",xml_file->getAttributeValueSafe(L"type")))
{
int index = cmbDisplayDriver->FindString("Direct3D 9 Renderer");
cmbDisplayDriver->SelectedIndex = index;
}
else
if(!std::wcscmp(L"EDT_OPENGL",xml_file->getAttributeValueSafe(L"type")))
{
int index = cmbDisplayDriver->FindString("OpenGL Renderer");
cmbDisplayDriver->SelectedIndex = index;
}
}
else if(!std::wcscmp(L"ScreenResHeight",xml_file->getNodeName()))
{
// save off screen resolution xml height value
std::wcscpy(formatted_height,xml_file->getAttributeValueSafe(L"value"));
}
else if(!std::wcscmp(L"ScreenResWidth",xml_file->getNodeName()))
{
// save off screen resolution xml width value
std::wcscpy(formatted_width,xml_file->getAttributeValueSafe(L"value"));
}
else if(!std::wcscmp(L"ScreenResBits",xml_file->getNodeName()))
{
// save off screen resolution xml bits value
std::wcscpy(formatted_bits,xml_file->getAttributeValueSafe(L"value"));
}
break;
// xml element
case io::EXN_ELEMENT_END:
{
// define array to hold a formatted screen resolution of the availabe modes
wchar_t formatted_screenresolution[64] = L" ";
// format available modes into desired listing
swprintf(
formatted_screenresolution,
64,
L"%s x %s pixels @ %s-bit colors",
formatted_height,
formatted_width,
formatted_bits);
// convert wchar_t to string object
System::String^ screen_res_xml_value = gcnew System::String(formatted_screenresolution);
// look for xml string in the list of drivers
int index = cmbScreenResolution->FindString(screen_res_xml_value);
// cannot find xml resolution in list of resolution index
if(index == -1)
{
// show popup saying unable to locate resolution in index
MessageBox::Show(
"No matching screen resolution found! Falling back to default settings",
"XML value for screen resolution cannot be found.",
MessageBoxButtons::OK,
MessageBoxIcon::Exclamation);
// set the combobox to show 1st resolution
cmbScreenResolution->SelectedIndex = 0;
}
// found xml resolution in list of resolution index
else
{
// set the combobox to show the found index to use as default driver
cmbScreenResolution->SelectedIndex = index;
}
}
break;
default:
break;
}
}
}
Update:
Download from here (uses rar; 557 KB):
http://www.megaupload.com/?d=JOBDDW2P
The buttons aren't working yet (close app using the "x" in the upper right of the program window), so you can try changing the xml file and see it load the values. It requires the .net 2.0 runtime. You can download it from here:
http://www.microsoft.com/downloads/deta ... laylang=en
Bye for now.
