- Can be dynamically sized.
- User settable grid spacing
- Can accent every nth line with a different color
- Can be scaled, rotated, ect like any other scene node.
- Everything set in the constructor can be later changed via SetFoo() and GetFoo() functions.
- Can Color Lines Along the Respective Axises.
- Has a clone() method
Notes:
Setting accentlineoffset to 0 causes accentlines to not be rendered.
If you need a grid on the XY or ZY axis, simply rotate this node by 90 degrees in the appropiate axis.
This node creates an XZ grid by default, which should be fine for normal use.
Usage:
Code: Select all
scene::CGridSceneNode* grid = new scene::CGridSceneNode(smgr->getRootSceneNode(), smgr);
grid->drop();
http://darkkilauea.deathtouchstudios.co ... neNode.zip
Screenshot:
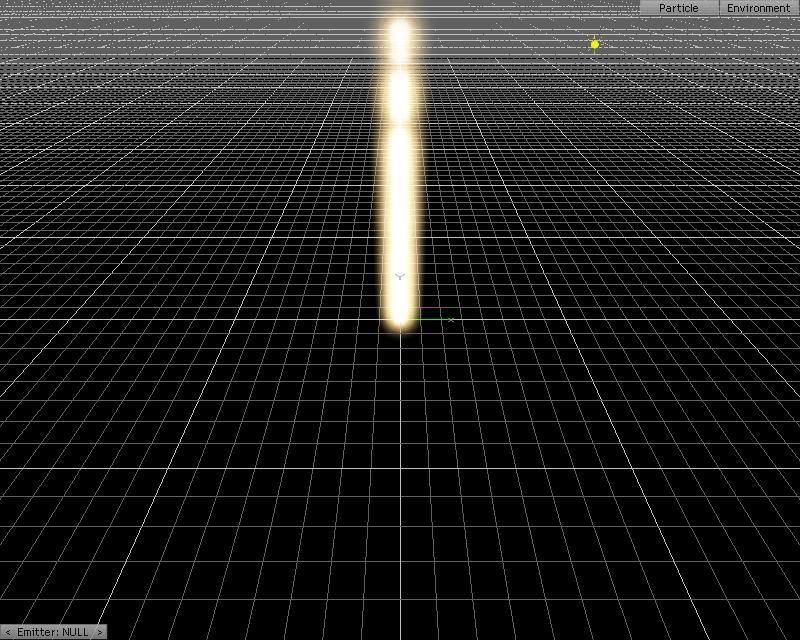
Final Note:
Mohaps' Grid class was very helpful in creating this node. Big thanks goes out to him.
[Update Sept. 27, 2009]
- Disabled z-writing for the grid to fix a flickering bug (thanks to ceyron)
[Update Aug. 21, 2009]
- Added a fix to a nasty crash bug by vins
[Update Oct. 23, 2008]
- Added JP's optimizations to the rendering process
- Put the node in the irr::scene namespace
- Modifed includes to only include what is necessary (less bloat)
- Renamed Clone() to clone() to fit with ISceneNode
- Grid can be regenerated using RegenerateGrid()
- Minor bug fixes
[Update Jan. 08, 2008]
- Code Cleaned up to work with Doxygen better
- Various bug fixes with wrong types and default variable values
- Culling now defaults to EAC_FRUSTUM_BOX
- Clone() now copies the material as well
[Update Jan. 07, 2008]
- Added Clone() method
- Fixed issue with signed integers, now the size, spacing, ect fields are u32.
- Culling defaults to EAC_BOX
[Update Jan. 04, 2008]
- Added new feature: Colored axis lines.
- Fixed floating point bug with BB Box.
- Cleaned up rendering code to speed things up under stress.