Help you me
Help you me
Excuse me but i from Italian and i speake italian, i excuse me for my bad english.
I have loaded a plane(mesh), and I would like to press left, keyboard, the mesh rotate step left, how can we do?
In Italian:
I ho caricato una mesh (aereo), e vorrei che alla pressione del tasto sinistro, della tastiera, la mesh ruotasse verso sinistra , come posso fare ?
Thanks e byby
I have loaded a plane(mesh), and I would like to press left, keyboard, the mesh rotate step left, how can we do?
In Italian:
I ho caricato una mesh (aereo), e vorrei che alla pressione del tasto sinistro, della tastiera, la mesh ruotasse verso sinistra , come posso fare ?
Thanks e byby
-
- Posts: 362
- Joined: Sun Dec 16, 2007 9:25 pm
use something like this: (replace "node" with your node)
(in the while(device->run()) loop)
You can change TurnTheNode.X to something like TurnTheNode.Z or even Y to change how it rotates
(in the while(device->run()) loop)
Code: Select all
vector3df TurnTheNode = node->getRotation();
TurnTheNode.X += 0.5f;
node->setRotation(TurnTheNode);
Post this userbar I made on other websites to show your support for Irrlicht!

http://img147.imageshack.us/img147/1261 ... wernq4.png

http://img147.imageshack.us/img147/1261 ... wernq4.png
twilight, how does that use the keyboard? 
Gianni, look at the tutorials/examples provided with the engine, the movement example should give you a good start here. But instead of moving the node when the button is pressed you can change the rotation instead (basically the same code, just need to change a couple of functions for rotation instead of position).

Gianni, look at the tutorials/examples provided with the engine, the movement example should give you a good start here. But instead of moving the node when the button is pressed you can change the rotation instead (basically the same code, just need to change a couple of functions for rotation instead of position).
Thank twilight17, i changed the code so:
But I wish that, as I leave the Left, the node stops in that position, where I left the key, I understand? I ask you how, and I thank you.[/code]
Code: Select all
.....
case KEY_LEFT:
{
if(event.KeyInput.PressedDown)
{
vector3df TurnTheNode = nPlane->getRotation();
TurnTheNode.Y -= 0.5f;
nPlane->setRotation(TurnTheNode);
nPlane->setPosition(core::vector3df(0,0,0));
}
if(!event.KeyInput.PressedDown)
return true;
.....
-
- Posts: 362
- Joined: Sun Dec 16, 2007 9:25 pm
Ahh I see what you're saying, you want it to stop in it position when you stop holding down the key?.. then add this above the int main()
This will allow smooth input with any key.
then At the start of main() right after the { put MyEventReceiver reveiver;
then, when you make the device use something like this: IrrlichtDevice *device = createDevice(EDT_OPENGL, dimension2d<s32>(640, 480), 16, false, false, false, &receiver);
Then in the while(device->run())
Adjust code accordingly 
Code: Select all
class MyEventReceiver : public IEventReceiver
{
public:
// This is the one method that we have to implement
virtual bool OnEvent(const SEvent& event)
{
// Remember whether each key is down or up
if (event.EventType == EET_KEY_INPUT_EVENT)
KeyIsDown[event.KeyInput.Key] = event.KeyInput.PressedDown;
return false;
}
// This is used to check whether a key is being held down
virtual bool IsKeyDown(EKEY_CODE keyCode) const
{
return KeyIsDown[keyCode];
}
MyEventReceiver()
{
for (u32 i=0; i<KEY_KEY_CODES_COUNT; ++i)
KeyIsDown[i] = false;
}
private:
// We use this array to store the current state of each key
bool KeyIsDown[KEY_KEY_CODES_COUNT];
};
then At the start of main() right after the { put MyEventReceiver reveiver;
then, when you make the device use something like this: IrrlichtDevice *device = createDevice(EDT_OPENGL, dimension2d<s32>(640, 480), 16, false, false, false, &receiver);
Then in the while(device->run())
Code: Select all
if(receiver.IsKeyDown(KEY_KEY_E))
{
vector3df turnit = node->getRotation();
turnit.X += 0.3f;
node->setRotation(turnit);
}

Post this userbar I made on other websites to show your support for Irrlicht!

http://img147.imageshack.us/img147/1261 ... wernq4.png

http://img147.imageshack.us/img147/1261 ... wernq4.png
Then I solved the problem so
Now remains one problem, I should done that the tecamera turn, based aircraft, but that is what must be the button is not pressed.
Code: Select all
#include <irrlicht.h>
#include <iostream>
using namespace irr;
using namespace core;
#pragma comment(lib, "Irrlicht.lib")
scene::ISceneNode* nPlane = 0;
IrrlichtDevice* device = 0;
int speed;
class MyEventReceiver : public IEventReceiver
{
public:
virtual bool OnEvent(const SEvent& event)
{
if(event.EventType == irr::EET_KEY_INPUT_EVENT)
{
switch(event.KeyInput.Key)
{
case KEY_ESCAPE:
{
device->closeDevice();
return true;
}
case KEY_LEFT:
{
if(event.KeyInput.PressedDown)
{
vector3df TurnTheNode = nPlane->getRotation();
TurnTheNode.Y -= 0.5f;
nPlane->setRotation(TurnTheNode);
}
if(!event.KeyInput.PressedDown)
;
return true;
}
case KEY_RIGHT:
{
if(event.KeyInput.PressedDown)
{
vector3df TurnTheNode = nPlane->getRotation();
TurnTheNode.Y += 0.5f;
nPlane->setRotation(TurnTheNode);
}
if(!event.KeyInput.PressedDown)
;
return true;
}
case KEY_UP:
{
if(event.KeyInput.PressedDown)
speed += 5;
if(!(event.KeyInput.PressedDown))
speed -= 100;
return true;
}
case KEY_DOWN:
{
speed -= 35;
return true;
}
case KEY_UP && KEY_LEFT:
{
nPlane->setPosition(core::vector3df(nPlane->getPosition().X,45.0,0));
core::vector3df newRot(0, 0.5, 0);
nPlane->setPosition(nPlane->getPosition());
return true;
}
default:
return false;
}
}
return false;
}
};
int main()
{
MyEventReceiver receiver;
device = createDevice( video::EDT_OPENGL, core::dimension2d<s32>(800, 600),
16, false, false, false, &receiver);
//IN CASO DI ERRORI IL PROGRAMMA VIENE TERMINATO
if (device == 0)
return 1;
video::IVideoDriver* driver = device->getVideoDriver();
scene::ISceneManager* smgr = device->getSceneManager();
//---------------------------------------------------------------------AEREO
device->getFileSystem()->addZipFileArchive("aereo.zip");
scene::IAnimatedMesh* mPlane = smgr->getMesh("aereo.3ds");
if (mPlane)
nPlane = smgr->addAnimatedMeshSceneNode(mPlane);
if (nPlane)
{
nPlane->setPosition(core::vector3df(0, 200, -1000));
nPlane->setMaterialFlag(video::EMF_LIGHTING, false);//METTO LA LUCE
}
//--------------------------------------------------------------------TEMPIO
device->getFileSystem()->addZipFileArchive("tempio.zip");
scene::IAnimatedMesh* mTemple = smgr->getMesh("tempio.dmf");
scene::ISceneNode* nTemple = 0;
if (mTemple)
nTemple = smgr->addAnimatedMeshSceneNode(mTemple);
if (nTemple)
{
nTemple->setPosition(core::vector3df(0, 0, 0));
nTemple->setMaterialFlag(video::EMF_LIGHTING, false);
}
//--------------------------------------------------------------------CAMERA
scene::ICameraSceneNode * camera = smgr->addCameraSceneNode();
camera->setPosition(core::vector3df(0, 50, -50));
device->getCursorControl()->setVisible(false);
speed = 0;
//-----------------------------------------------------------------MAIN LOOP
while(device->run())
{
driver->beginScene(true, true, video::SColor(0,200,200,200));
smgr->drawAll();
driver->endScene();
core::stringw str = L" ";
str += " AEREO";
str += " SPEED: ";
str += speed;
str += " Km/h";
device->setWindowCaption(str.c_str());
//-------------------------------------------------------SET POSITION PLANE
if(speed >2000)
speed = 2000;
if(speed < 0)
speed = 0;
float angle = nPlane->getRotation().Y; // legge al posizione sull'asse Y
core::vector3df newPos(0,0,(5*speed)/250);
nPlane->setPosition(nPlane->getPosition() + newPos);
//-----------------------------------------------------------SET CAMERA
angle = angle + 90;
newPos = nPlane->getPosition();
core::vector3df newTarg = newPos;
newPos.X = newPos.X + ( cos( angle * 3.14159 / 180) * 50);
newPos.Z = newPos.Z + ( sin( angle * 3.14159 / 180) * -50);
newPos.Y = newPos.Y + 50;
camera->setPosition(newPos);
camera->setRotation(nPlane->getRotation());
camera->updateAbsolutePosition();
newTarg.Y = newTarg.Y + 50;
camera->setTarget(newTarg);
camera->updateAbsolutePosition();
}
device->drop();
return 0;
}
Now remains one problem, I should done that the tecamera turn, based aircraft, but that is what must be the button is not pressed.
-
- Posts: 362
- Joined: Sun Dec 16, 2007 9:25 pm
Ahhh nooo, you dont put the key input there, you do this: (everything is alright so far, just replace your event receiver with the one I posted earlier)Gianni wrote:Code: Select all
class MyEventReceiver : public IEventReceiver { public: virtual bool OnEvent(const SEvent& event) { if(event.EventType == irr::EET_KEY_INPUT_EVENT) { [b]switch(event.KeyInput.Key) { case KEY_ESCAPE: { device->closeDevice(); return true; } case KEY_LEFT: { if(event.KeyInput.PressedDown) { vector3df TurnTheNode = nPlane->getRotation(); TurnTheNode.Y -= 0.5f; nPlane->setRotation(TurnTheNode); } if(!event.KeyInput.PressedDown) ; return true; } case KEY_RIGHT: { if(event.KeyInput.PressedDown) { vector3df TurnTheNode = nPlane->getRotation(); TurnTheNode.Y += 0.5f; nPlane->setRotation(TurnTheNode); } if(!event.KeyInput.PressedDown) ; return true; } case KEY_UP: { if(event.KeyInput.PressedDown) speed += 5; if(!(event.KeyInput.PressedDown)) speed -= 100; return true; } case KEY_DOWN: { speed -= 35; return true; } case KEY_UP && KEY_LEFT: { nPlane->setPosition(core::vector3df(nPlane->getPosition().X,45.0,0)); core::vector3df newRot(0, 0.5, 0); nPlane->setPosition(nPlane->getPosition()); return true; } default: return false; }[/b] } return false; } };
Code: Select all
int main()
{
(your code)
while (device->run())
{
if (receiver.IsKeyDown(KEY_LEFT) //change this to whatever key you need
{
vector3df TurnTheNode = node->getRotation();
TurnTheNode.X += 0.5f;
node->setRotation(TurnTheNode);
(your other code)
}

Post this userbar I made on other websites to show your support for Irrlicht!

http://img147.imageshack.us/img147/1261 ... wernq4.png

http://img147.imageshack.us/img147/1261 ... wernq4.png
ok thank to all. Now I would like to put collision between the plane and the temple. But I do not know anything about collisions,and someone I can say those types of collisions we? What types? Which should I use in my case? And how?
In Italian:
OK grazie a tutti. Ora i vorrei mettere una collsione sul tempio e l'aereo.
Ma io non so niente sulle collisioni, quindi qualcuno mi puo dire quanti tipi di collisioni abbiamo ? Quali tipi ? Quale dovrei usare nel mio caso ? e come ?
In Italian:
OK grazie a tutti. Ora i vorrei mettere una collsione sul tempio e l'aereo.
Ma io non so niente sulle collisioni, quindi qualcuno mi puo dire quanti tipi di collisioni abbiamo ? Quali tipi ? Quale dovrei usare nel mio caso ? e come ?
-
- Posts: 362
- Joined: Sun Dec 16, 2007 9:25 pm
Why would you put collision between the plane and the temple, wouldnt it be from the hero to the plane or temple? Explain please 

Post this userbar I made on other websites to show your support for Irrlicht!

http://img147.imageshack.us/img147/1261 ... wernq4.png

http://img147.imageshack.us/img147/1261 ... wernq4.png
I did not put any collsione. Let me know how you make the collsioni? The reason is as follows: With the plane step all over the walls, and is not good in a game, move walls, roofs, floors, and objects.
In italian:
Io non ho messo alcuna collsione. Vorrei sapere come si mettono le collsioni ? Il motivo è il seguente : Con l'aereo passo tutti i muri, e non è bello in un gioco, passare muri, tetti, pavimenti, e oggetti.
In italian:
Io non ho messo alcuna collsione. Vorrei sapere come si mettono le collsioni ? Il motivo è il seguente : Con l'aereo passo tutti i muri, e non è bello in un gioco, passare muri, tetti, pavimenti, e oggetti.
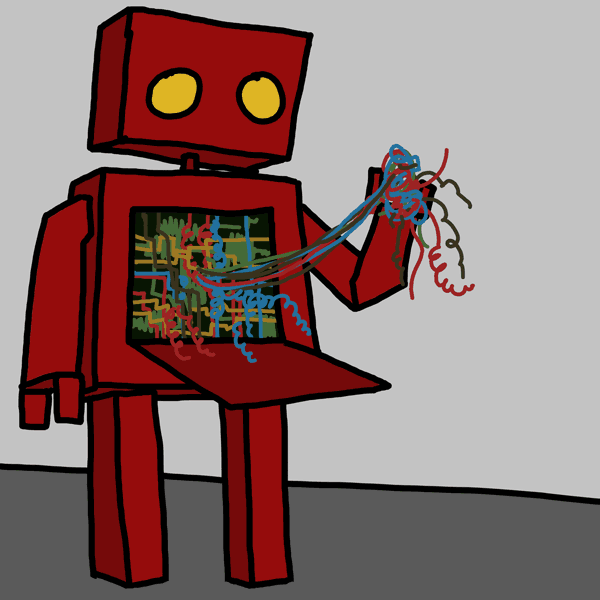
DOES NOT COMPUTE!
Is your problem that you want your camera/character to collide with BOTH the plane and the temple, and at the moment you can only get it to collide with either the plane OR the temple?
If that's the case then look at the IMetaTriangleSelector. You can create one of these (using smgr->createMetaTriangleSelector()) and then add BOTH the plane triangle selector and the temple triangle selector and then passing that meta triangle selector to the collision response animator (if that's what you're using).
Re: Help you me
Yes. i have the similar problem.Gianni wrote:Excuse me but i from Italian and i speake italian, i excuse me for my bad english.
I have loaded a plane(mesh), and I would like to press left, keyboard, the mesh rotate step left, how can we do?
In Italian:
I ho caricato una mesh (aereo), e vorrei che alla pressione del tasto sinistro, della tastiera, la mesh ruotasse verso sinistra , come posso fare ?
Thanks e byby
I love age of conan gold and age of conan powerleveling