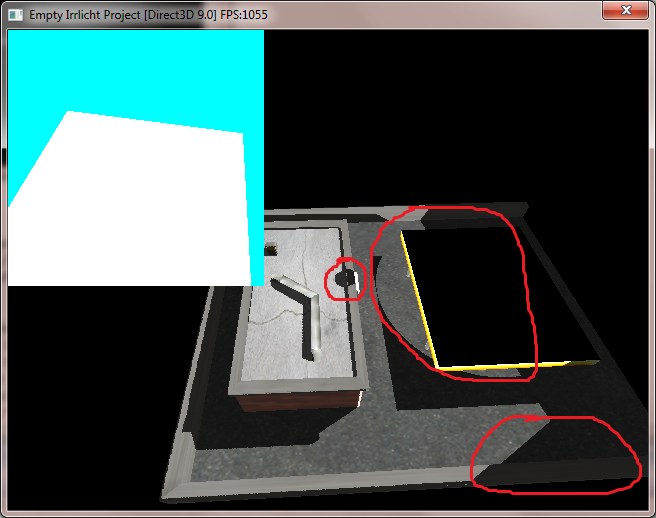
Shadow Map:
Code: Select all
float4x4 LightsWorldViewProjection;
float4x4 WorldViewProjection;
float4x4 World;
float3 LightPos;
float distanceScale = 0.4;
struct VS_OUTPUT {
float4 Pos: POSITION;
float3 lightVec: TEXCOORD0;
};
VS_OUTPUT vertexMain(float4 Pos: POSITION){
VS_OUTPUT Out;
float4 pos;
Out.Pos = mul(Pos,LightsWorldViewProjection);
Pos.xyz -= LightPos;
Out.lightVec = distanceScale * Pos;
return Out;
}
float4 pixelMain(float3 lightVec: TEXCOORD0) : COLOR {
// Output radial distance
float4 output = 0;
output = length(lightVec);
return output;
}
Code: Select all
float4x4 LightsWorldViewProjection;
float4x4 WorldViewProjection;
float3 LightPos;
float Ambient;
float distanceScale = 0.4;
sampler2D tex0;
sampler2D tex1;
struct VS_OUTPUT {
float4 Pos: POSITION;
float2 texCoord: TEXCOORD0;
float3 normal: TEXCOORD1;
float3 lightVec : TEXCOORD2;
float4 shadowCrd: TEXCOORD3;
};
VS_OUTPUT vertexMain(float2 texCoord: TEXCOORD0, float4 Pos: POSITION, float3 normal: NORMAL){
VS_OUTPUT Out;
Out.Pos = mul( Pos, WorldViewProjection);
Out.normal = normal;
Out.lightVec = distanceScale * ( Pos.xyz-LightPos );
Out.texCoord = texCoord;
Pos.xyz -= LightPos;
float4 sPos = mul( Pos, LightsWorldViewProjection);
Out.shadowCrd.x = 0.5 * (sPos.z + sPos.x);
Out.shadowCrd.y = 0.5 * (sPos.z - sPos.y);
Out.shadowCrd.z = 0;
Out.shadowCrd.w = sPos.z;
return Out;
}
float4 pixelMain(float2 texCoord : TEXCOORD0, float3 normal: TEXCOORD1, float3 lightVec: TEXCOORD2, float4 shadowCrd: TEXCOORD3) : COLOR {
normal = normalize(normal);
float depth = length(lightVec);
float4 base = tex2D(tex0, texCoord);
float shadowMap = tex2Dproj(tex1, shadowCrd).r;
float shadow = (depth < shadowMap);
return shadow*base+base*Ambient;
}