Instead of rendering EPT_QUADS I'm getting 3d lines that look like they span the aabbox extents?
I've carefully ensured that my Project Properties are all the same for both builds, including the same Directories, etc.
Very odd, I'm not sure where to begin debugging this?
Debug build: ( how it should look)
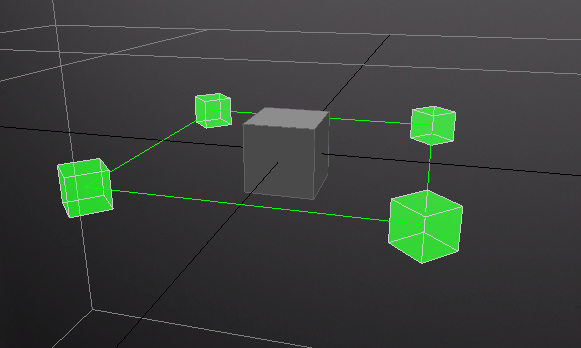
Release build: ??
The gray square is rendering correctly because it is an irr::scene::CCubeSceneNode
Only my custom cubes are not rendering correctly
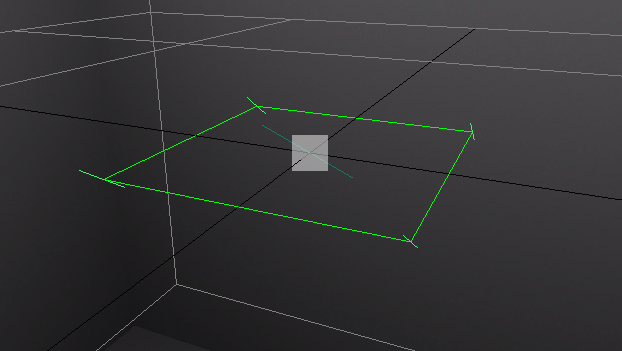
Perhaps it has something to do with the way I've designed my custom cubes:
Code: Select all
class kxCubeNode : public ISceneNode
{
public:
//! constructor
kxCubeNode();
virtual ~kxCubeNode() {}
virtual void OnRegisterSceneNode();
virtual void render();
virtual const core::aabbox3d<f32>& getBoundingBox() const { return m_box; }
virtual video::SMaterial& getMaterial( u32 i ) { return m_material; }
virtual video::SMaterial& getEdgeMaterial() { return m_edgeMat; }
virtual u32 getMaterialCount() const { return 1; }
virtual ESCENE_NODE_TYPE getType() const { return (ESCENE_NODE_TYPE) KNT_CUBE; }
virtual void makeMesh(); // creates a new unit cube
virtual void setSize( const f32 newSize ); // multiplies all vertices positions by newSize
f32 getSize() { return m_size; }
virtual void setPosition( const vector3df& pos ) { ISceneNode::setPosition( pos ); }
bool m_showNormals;
f32 m_size;
SMaterial m_material;
SMaterial m_edgeMat;
core::aabbox3d<f32> m_box;
array< S3DVertex > m_vertices;
array< u16 > m_indices;
array< u16 > m_edgeIndices;
kx& k; // context
};
kxCubeNode::kxCubeNode():
k( kx::getInstance() ),
ISceneNode( kx::getInstance().smgr->getRootSceneNode(), kx::getInstance().smgr, KNT_CUBE ),
m_size( 1.6f ), m_showNormals( false )
{
#ifdef _DEBUG
setDebugName( "kxCubeNode" );
#endif
setName( "kxCubeNode" );
m_material.setTexture( 0, k.grayTex );
m_material.Lighting= true;
m_material.GouraudShading= false;
m_edgeMat.Lighting= false;
makeMesh();
setSize( m_size );
}
void kxCubeNode::makeMesh()
{
/*
* 24 vertices; each face gets it's own 4 with normals
* rendered with EPT_QUADS
5.8.18-----------3.11.19
/| /| +y
/ | / | ^ +z
/ | / | | /
4.9.22-----------0.10.21 | /
| | | | |/
6.14.17-------|--2.15.16 *----->+x
| / | /
| / | /
|/ |/
7.13.23-----------1.12.20
*/
// vertex array
f32 s= m_size/2;
const f32 va[192] =
// Pos Normals TCoords Vertex Face
{
s, s, -s, 1, 0, 0, 0, 0, // 0 +x
s, -s, -s, 1, 0, 0, 0, 1, // 1
s, -s, s, 1, 0, 0, 1, 1, // 2
s, s, s, 1, 0, 0, 1, 0, // 3
-s, s, -s, -1, 0, 0, 1, 0, // 4 -x
-s, s, s, -1, 0, 0, 0, 0, // 5
-s, -s, s, -1, 0, 0, 0, 1, // 6
-s, -s, -s, -1, 0, 0, 1, 1, // 7
-s, s, s, 0, 1, 0, 0, 0, // 8 +y
-s, s, -s, 0, 1, 0, 0, 1, // 9
s, s, -s, 0, 1, 0, 1, 1, // 10
s, s, s, 0, 1, 0, 1, 0, // 11
s, -s, -s, 0, -1, 0, 0, 1, // 12 -y
-s, -s, -s, 0, -1, 0, 1, 1, // 13
-s, -s, s, 0, -1, 0, 1, 0, // 14
s, -s, s, 0, -1, 0, 0, 0, // 15
s, -s, s, 0, 0, 1, 0, 1, // 16 +z
-s, -s, s, 0, 0, 1, 1, 1, // 17
-s, s, s, 0, 0, 1, 1, 0, // 18
s, s, s, 0, 0, 1, 0, 0, // 19
s, -s, -s, 0, 0, -1, 1, 1, // 20 -z
s, s, -s, 0, 0, -1, 1, 0, // 21
-s, s, -s, 0, 0, -1, 0, 0, // 22
-s, -s, -s, 0, 0, -1, 0, 1 // 23
};
// Vertices
video::S3DVertex vx;
//vx.Color.set(255,255,255,255);
u32 i=0;
for( s32 j=0; j<24; j++ )
{
vx.Pos.set( vector3df( va[i++], va[i++], va[i++] ));
vx.Normal.set( vector3df( va[i++], va[i++], va[i++] ));
vx.TCoords.set( vector2df( va[i++], va[i++] ));
m_vertices.push_back( vx );
}
// quad indices
for (u16 k=0; k<24; k++)
m_indices.push_back( k );
m_box= aabbox3df( m_vertices[0].Pos, m_vertices[6].Pos );
// edge indices
const u16 ei[24] = { 0,1, 1,2, 2,3, 3,0, 4,5, 5,6, 6,7, 7,4, 0,4, 1,7, 2,6, 3,5 };
for (u16 e=0; e<24; e++)
m_edgeIndices.push_back( ei[e] );
}
void kxCubeNode::setSize( const f32 newSize )
{
for( u32 vx= 0; vx<m_vertices.size(); vx++ )
{
m_vertices[vx].Pos *= vector3df( newSize/m_size );
//m_vertices[vx].Normal *= vector3df( m_size/2 );
}
m_size= newSize;
}
void kxCubeNode::render()
{
k.driver->setMaterial( m_material );
k.driver->setTransform( ETS_WORLD, AbsoluteTransformation );
k.driver->drawVertexPrimitiveList(
&m_vertices[0], m_vertices.size(),
&m_indices[0],
6, // primitive count
EVT_STANDARD, EPT_QUADS,
EIT_16BIT
);
// DRAW EDGES
// build nudge matrix to avoid z-fighting
vector3df camV= SceneManager->getActiveCamera()->getAbsolutePosition() - RelativeTranslation;
f32 length= .01f;
f32 scale= ( camV.getLength() - length ) / camV.getLength();
camV.setLength( length );
camV += RelativeTranslation;
matrix4 mx;
mx.setScale( scale * RelativeScale );
mx.setRotationDegrees( RelativeRotation );
mx.setTranslation( camV );
k.driver->setTransform( ETS_WORLD, mx );
k.driver->setMaterial( m_edgeMat );
k.driver->drawVertexPrimitiveList( &m_vertices[0], m_vertices.size(), &m_edgeIndices[0], 12, EVT_STANDARD, EPT_LINES );
if( m_showNormals )
for( u32 vx= 0; vx<m_vertices.size(); vx++ )
k.driver->draw3DLine
(
m_vertices[vx].Pos,
m_vertices[vx].Pos + m_vertices[vx].Normal,
SColor( 255,255,0,255 ) // purple
);
}