Maths not being my strong point I wondering how to move an object from A to B on a parabolic trajectory.
eg
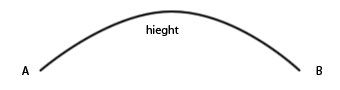
I know if I was in 2D to make x,y get to c,b I would just have to increase x, or y until it reaches coordinate c,b over time.
First of all I have been moving things around in irrlicht by trial and error. I know you have x,y and z coordinates, and I know the middle coordinate is height.
In 3ds max, you have x and y, and z is height.
In irrlicht I have worked out that this would be (0,20,0) hieght, but I am not sure if that middle coordinate is z in irrlicht.
Anyway to get a piece moving on a parabola I would need to increase hieght, over time, and when it gets to the centre, decrease hieght.
While doing this x and y would also be moving towards their target.
X and Y are easy to compute ish, but z being hieght would be very hard to work out. Well unless you are very good with forumulas.
Any ideas?
I presume this is complex as I can't use loops. I suspect a formula would require checking for if the piece has reached the centre and then switch it about so that it descends towards the correct hieght.