Unfortunately, I project I'm working with is stuck with Irrlicht's built-in GUIs until we get around to making a replacement. I'd like to add the ability to dynamically style elements.
Working picture showing you what I mean:
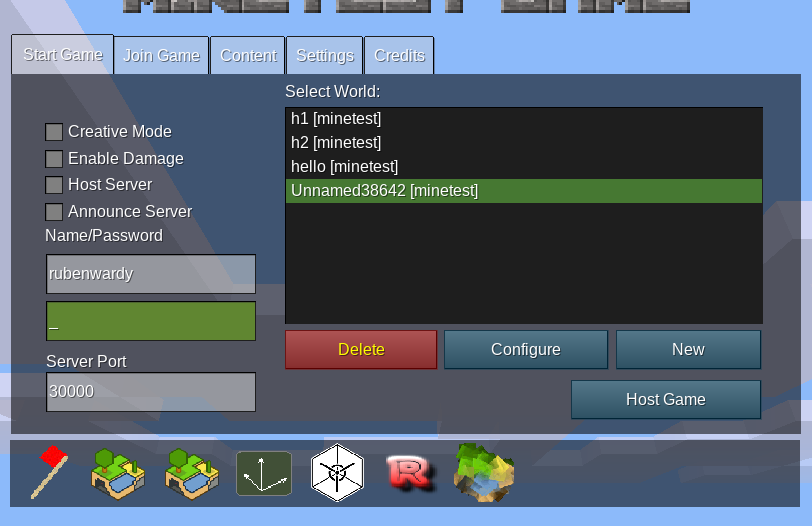
I've copied CGUIButton and CGUISKin to my project.
I had to do the former because you don't allow subclassing due to the inaccessible headers for CGUIButton, which is incredibly annoying.
I had to do the latter to implement a drawColored3DButtonStandard and drawColored3DButtonPressed, but I may try moving these functions into CGUIButton itself to reduce the risk of breakages.
Is there a better way of doing this?
I've effectively backported a few of the button improvements including override colors, which means that I have to have ugly code like this:
Code: Select all
#if (IRRLICHT_VERSION_MAJOR > 1 || IRRLICHT_VERSION_MINOR != 8 || IRRLICHT_VERSION_REVISION < 5)
namespace irr { namespace gui {
//! State of buttons used for drawing texture images.
//! Note that only a single state is active at a time
//! Also when no image is defined for a state it will use images from another state
//! and if that state is not set from the replacement for that,etc.
//! So in many cases setting EGBIS_IMAGE_UP and EGBIS_IMAGE_DOWN is sufficient.
enum EGUI_BUTTON_IMAGE_STATE {
//! When no other states have images they will all use this one.
EGBIS_IMAGE_UP,
//! When not set EGBIS_IMAGE_UP is used.
EGBIS_IMAGE_UP_MOUSEOVER,
//! When not set EGBIS_IMAGE_UP_MOUSEOVER is used.
EGBIS_IMAGE_UP_FOCUSED,
//! When not set EGBIS_IMAGE_UP_FOCUSED is used.
EGBIS_IMAGE_UP_FOCUSED_MOUSEOVER,
//! When not set EGBIS_IMAGE_UP is used.
EGBIS_IMAGE_DOWN,
//! When not set EGBIS_IMAGE_DOWN is used.
EGBIS_IMAGE_DOWN_MOUSEOVER,
//! When not set EGBIS_IMAGE_DOWN_MOUSEOVER is used.
EGBIS_IMAGE_DOWN_FOCUSED,
//! When not set EGBIS_IMAGE_DOWN_FOCUSED is used.
EGBIS_IMAGE_DOWN_FOCUSED_MOUSEOVER,
//! When not set EGBIS_IMAGE_UP or EGBIS_IMAGE_DOWN are used (depending on button state).
EGBIS_IMAGE_DISABLED,
//! not used, counts the number of enumerated items
EGBIS_COUNT
};
//! Names for gui button image states
const c8 *const GUIButtonImageStateNames[EGBIS_COUNT + 1] =
{
"Image", // not "ImageUp" as it otherwise breaks serialization of old files
"ImageUpOver",
"ImageUpFocused",
"ImageUpFocusedOver",
"PressedImage", // not "ImageDown" as it otherwise breaks serialization of old files
"ImageDownOver",
"ImageDownFocused",
"ImageDownFocusedOver",
"ImageDisabled",
0 // count
};
}}
#endif
Here's the link to the code: https://github.com/minetest/minetest/pull/8383
TL;DR: how would you recommend extending GUI elements to support more draw features?