https://sourceforge.net/p/irrlicht/code ... r.cpp#l898A cone with proper normals and texture coords.
[fixed]No texture coordinates in cone mesh
-
- Posts: 1186
- Joined: Fri Dec 29, 2006 12:04 am
[fixed]No texture coordinates in cone mesh
The CGeometryCreator creates cone meshes without texture coordinates despite the comment for CGeometryCreator::createConeMesh says so:
"Whoops..."
Re: No texture coordinates in cone mesh
Thanks. Thought not sure what good uv's would be. I'll take a look at it, but likely after X-Mas (gonna be without my PC for a few days...).
IRC: #irrlicht on irc.libera.chat
Code snippet repository: https://github.com/mzeilfelder/irr-playground-micha
Free racer made with Irrlicht: http://www.irrgheist.com/hcraftsource.htm
Code snippet repository: https://github.com/mzeilfelder/irr-playground-micha
Free racer made with Irrlicht: http://www.irrgheist.com/hcraftsource.htm
Re: No texture coordinates in cone mesh
Played around a bit with it and this seems to get close (assuming some radial texture):
But made a quick test and still getting ugly black artifacts. Not sure yet if those come from bad normals (maybe because side and bottom polygons share vertices) or because cone is not a real circle or bad test texture I created. I will get back to it next week probably. Maybe someone else has an idea in the meantime what I do wrong.
Test:
Current result (left-top the texture I use): 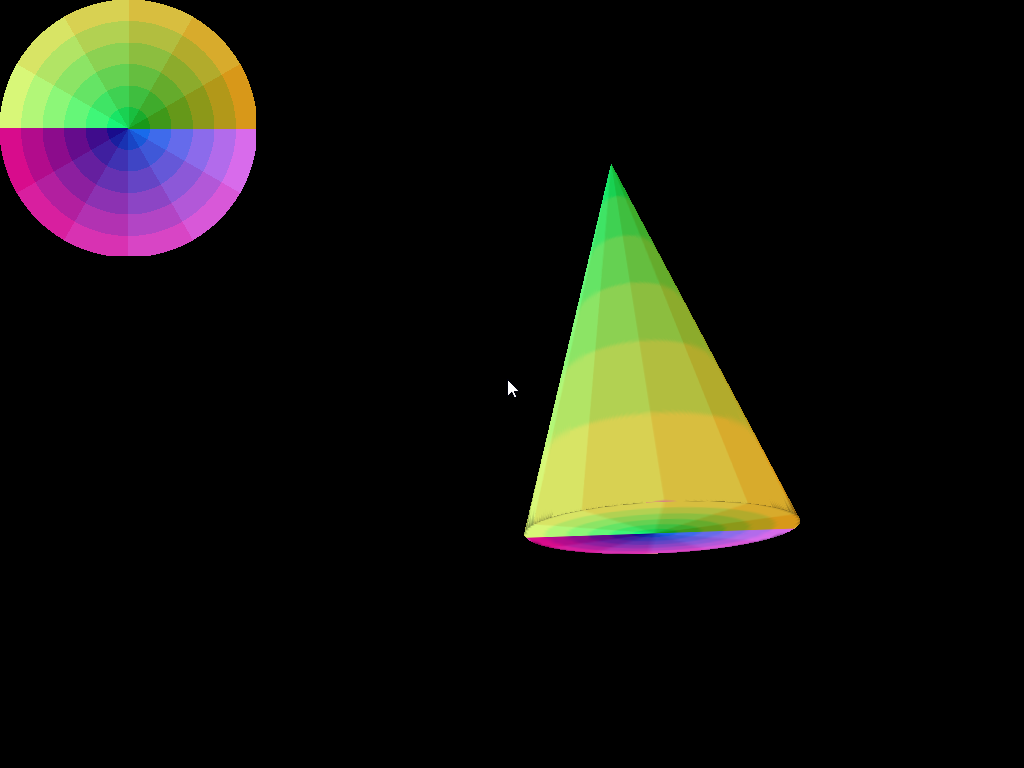
Code: Select all
// for those inside loop in CGeometryCreator::createConeMesh
v.TCoords.X = (1.f+cosf(angle))*0.5f;
v.TCoords.Y = (1.f+sinf(angle))*0.5f;
// after loop
v.TCoords.X = 0.5f;
v.TCoords.Y = 0.5f;
Test:
Code: Select all
#include <irrlicht.h>
using namespace irr;
using namespace core;
#ifdef _MSC_VER
#pragma comment(lib, "Irrlicht.lib")
#endif
core::dimension2du dim(1024, 1024);
irr::video::ITexture* addRadialTexture(irr::video::IVideoDriver* driver)
{
irr::video::ITexture* result = 0;
vector2df center(dim.Width*0.5f, dim.Height*0.5f);
f32 radius = core::min_(center.X, center.Y);
radius += 4; // reducing black gaps... still doing/understanding something wrong I suppose
const u32 bytesPerPixel = 4;
u8* data = new u8[dim.Width * dim.Height * bytesPerPixel];
const irr::u32 pitch = dim.Width * bytesPerPixel;
u32 stepsOutwards = 6;
u32 stepsCircular = 12;
for (u32 y = 0; y < dim.Height; ++y)
{
const u32 lineStart = y * pitch;
for (u32 x = 0; x < dim.Width; ++x)
{
u32 idx = lineStart + x*bytesPerPixel;
vector2df offset((f32)x-center.X, (f32)y-center.Y);
f32 offsetLen = offset.getLength();
if ( offsetLen > radius )
{
data[idx] = 0;
data[idx+1] = 0;
data[idx+2] = 0;
data[idx+3] = 0;
}
else
{
int circleStep = (int)(offset.getAngle()*(float)stepsCircular/360.f);
int outwardStep = (int)(offsetLen*(float)stepsOutwards/radius);
data[idx] = 25+circleStep*230/stepsCircular; // blue
data[idx+1] = (data[idx]+127)%255; // green
data[idx+2] = 25+outwardStep*230/stepsOutwards; // red
data[idx+3] = 255; // alpha
}
}
}
video::IImage* img = driver->createImageFromData(irr::video::ECF_A8R8G8B8, dim, data);
if (img)
{
result = driver->addTexture("radialCheckerboard", img);
img->drop();
}
delete[] data;
return result;
}
int main()
{
IrrlichtDevice * Device = createDevice(irr::video::EDT_OPENGL, irr::core::dimension2d<irr::u32>(1024,768));
if (!Device)
return false;
scene::ISceneManager* smgr = Device->getSceneManager();
video::IVideoDriver * videoDriver = Device->getVideoDriver ();
scene::IMesh* coneMesh = smgr->getGeometryCreator()->createConeMesh(50.f, 200.f, 60, irr::video::SColor(255, 255, 255, 255), irr::video::SColor(255, 255, 255, 255), 0.f);
coneMesh->getMeshBuffer(0)->getMaterial().Lighting = false;
video::ITexture* tex = addRadialTexture(videoDriver);
coneMesh->getMeshBuffer(0)->getMaterial().setTexture(0, tex);
smgr->addMeshSceneNode(coneMesh);
coneMesh->drop();
irr::scene::ICameraSceneNode * camera = smgr->addCameraSceneNodeFPS(0, 20.f, 0.1f );
camera->updateAbsolutePosition();
camera->setTarget(irr::core::vector3df(0,0,0));
camera->updateAbsolutePosition();
camera->setPosition(irr::core::vector3df(0, -150, -300));
camera->updateAbsolutePosition();
while ( Device->run() )
{
if ( Device->isWindowActive() )
{
videoDriver->beginScene(true, true);
smgr->drawAll();
recti destRect(0,0,dim.Width/4,dim.Height/4);
recti sourceRect(0,0,dim.Width,dim.Height);
videoDriver->draw2DImage(tex, destRect, sourceRect, 0, 0, true);
videoDriver->endScene();
}
Device->sleep(0);
}
Device->closeDevice();
Device->drop();
Device = NULL;
return 0;
}
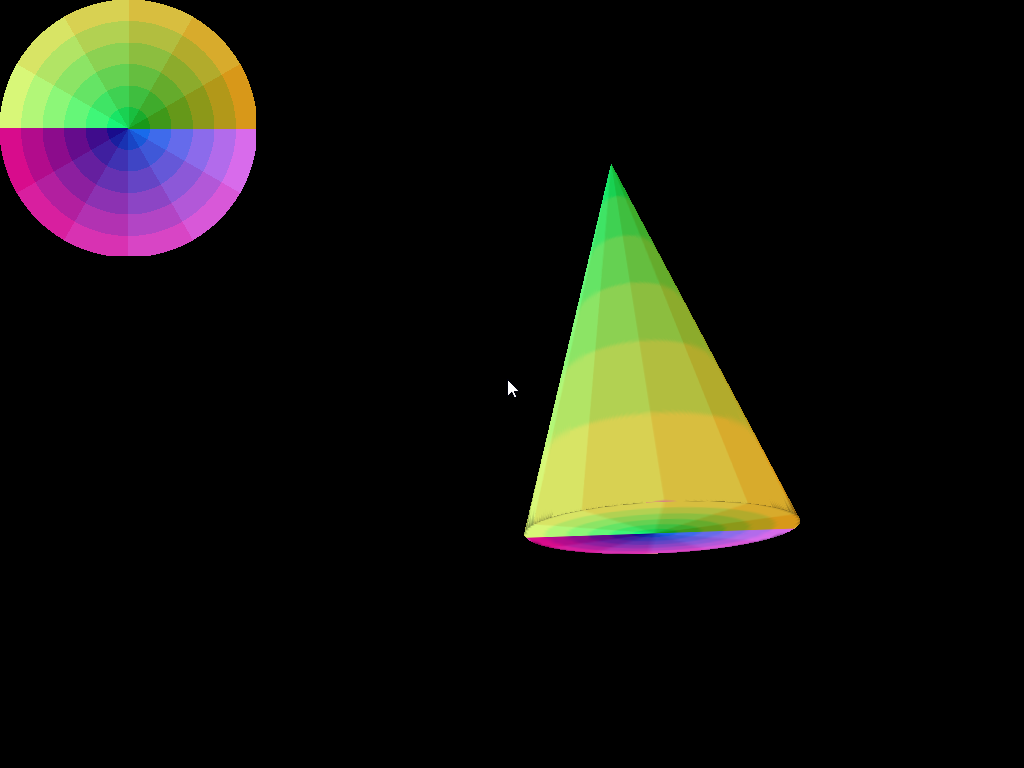
IRC: #irrlicht on irc.libera.chat
Code snippet repository: https://github.com/mzeilfelder/irr-playground-micha
Free racer made with Irrlicht: http://www.irrgheist.com/hcraftsource.htm
Code snippet repository: https://github.com/mzeilfelder/irr-playground-micha
Free racer made with Irrlicht: http://www.irrgheist.com/hcraftsource.htm
-
- Posts: 1186
- Joined: Fri Dec 29, 2006 12:04 am
Re: No texture coordinates in cone mesh
Hey,
thanks for looking into it.
Can't see your image, my browser shows a "broken image" icon.
I've applied your changes and this is what it looks like with a square texture:
https://imgur.com/a/rptS79q
Note that i don't use Irrlicht but only the mesh geometry code in a vanilla OpenGL app.
For me that's an improvement, so thank you again!
thanks for looking into it.
Can't see your image, my browser shows a "broken image" icon.
I've applied your changes and this is what it looks like with a square texture:
https://imgur.com/a/rptS79q
Note that i don't use Irrlicht but only the mesh geometry code in a vanilla OpenGL app.
For me that's an improvement, so thank you again!
"Whoops..."
Re: No texture coordinates in cone mesh
Strange no idea why image won't show up for you. But same on imgur: https://imgur.com/a/wVXSCwD
IRC: #irrlicht on irc.libera.chat
Code snippet repository: https://github.com/mzeilfelder/irr-playground-micha
Free racer made with Irrlicht: http://www.irrgheist.com/hcraftsource.htm
Code snippet repository: https://github.com/mzeilfelder/irr-playground-micha
Free racer made with Irrlicht: http://www.irrgheist.com/hcraftsource.htm
-
- Posts: 1186
- Joined: Fri Dec 29, 2006 12:04 am
Re: No texture coordinates in cone mesh
You seem to use an invalid SSL certificate on your server: https://imgur.com/a/zREL4A8Strange no idea why image won't show up for you.
Yes, the artifacts look weird. But the UVs are good i think, at least i don't see them in my app: https://imgur.com/a/ZTWHUlR
"Whoops..."
Re: No texture coordinates in cone mesh
Uh, OK - your browser updates links automatically to https when they are inside another https page. But that server does only have http. Anyway good to know this is a problem, guess I'll use imgur from now on in the forum.
The artifacts should be because it accesses things slightly outside the colored circle in my texture. Which it shouldn't unless I messed up something (I already increased the colored circle slightly). But I'll check again next week.
The artifacts should be because it accesses things slightly outside the colored circle in my texture. Which it shouldn't unless I messed up something (I already increased the colored circle slightly). But I'll check again next week.
IRC: #irrlicht on irc.libera.chat
Code snippet repository: https://github.com/mzeilfelder/irr-playground-micha
Free racer made with Irrlicht: http://www.irrgheist.com/hcraftsource.htm
Code snippet repository: https://github.com/mzeilfelder/irr-playground-micha
Free racer made with Irrlicht: http://www.irrgheist.com/hcraftsource.htm
-
- Posts: 1186
- Joined: Fri Dec 29, 2006 12:04 am
Re: No texture coordinates in cone mesh
I am using
on latest Raspberry PI OSChromium Version 120.0.6099.102 (Official Build) Built on Debian , running on Debian 12 (64-bit)
"Whoops..."
Re: No texture coordinates in cone mesh
Yeah, looks like it's like that in most browser by now since a while. Firefox being the usual exception. You can no longer use http images inside https pages. I really have to give another shot some day at pursuading support for whoever is my provider now to update my stuff. You can get some strange setups if your websites survives longer than your provider (provider got sold several times since I created my website...). It's even still on PHP 4 ;-) (I don't use PHP there so don't care).
IRC: #irrlicht on irc.libera.chat
Code snippet repository: https://github.com/mzeilfelder/irr-playground-micha
Free racer made with Irrlicht: http://www.irrgheist.com/hcraftsource.htm
Code snippet repository: https://github.com/mzeilfelder/irr-playground-micha
Free racer made with Irrlicht: http://www.irrgheist.com/hcraftsource.htm
Re: No texture coordinates in cone mesh
Changed now in svn r6580. Black parts in UV came from mipmaps in my case. Tiny discoloration is still there, but giving up on that.
And as usual when looking at code I found a bunch of other stuff *sigh*.
- Tesselation is for semi-circle instead of circle (so we can't have odd-numbered tesselation for cones and cylinders). Not sure how to fix that without breaking old code (add another parameter maybe to allow tesselation for full circle? Or just break? Or ignore?).
- Code strangely complicated (still the case for cylinders).
- I improved UV's for cylinder slightly. But would need to duplicate a few vertices for really nice results (bottom and top having the problem that Y is fixed due to sharing vertices with sides).
- Same for cone - right now UV's for bottom are kinda ok - the other have mirrored uv's (noticeable with text in texture)
- Cylinders also have mirrored UV's
I'll ignore all that for now and consider the bug fixed.
And as usual when looking at code I found a bunch of other stuff *sigh*.
- Tesselation is for semi-circle instead of circle (so we can't have odd-numbered tesselation for cones and cylinders). Not sure how to fix that without breaking old code (add another parameter maybe to allow tesselation for full circle? Or just break? Or ignore?).
- Code strangely complicated (still the case for cylinders).
- I improved UV's for cylinder slightly. But would need to duplicate a few vertices for really nice results (bottom and top having the problem that Y is fixed due to sharing vertices with sides).
- Same for cone - right now UV's for bottom are kinda ok - the other have mirrored uv's (noticeable with text in texture)
- Cylinders also have mirrored UV's
I'll ignore all that for now and consider the bug fixed.
IRC: #irrlicht on irc.libera.chat
Code snippet repository: https://github.com/mzeilfelder/irr-playground-micha
Free racer made with Irrlicht: http://www.irrgheist.com/hcraftsource.htm
Code snippet repository: https://github.com/mzeilfelder/irr-playground-micha
Free racer made with Irrlicht: http://www.irrgheist.com/hcraftsource.htm
Re: [fixed]No texture coordinates in cone mesh
OK, I decided I'll break existing code and fix the tessellation numbers. And cleaned up the code some more as well. UV's still not perfect, but at least we can now create stuff like triangle pyramids which was impossible before. Done in svn r6581.
Last commit for 2023 - Happy New Year everyone ;-)
Last commit for 2023 - Happy New Year everyone ;-)
IRC: #irrlicht on irc.libera.chat
Code snippet repository: https://github.com/mzeilfelder/irr-playground-micha
Free racer made with Irrlicht: http://www.irrgheist.com/hcraftsource.htm
Code snippet repository: https://github.com/mzeilfelder/irr-playground-micha
Free racer made with Irrlicht: http://www.irrgheist.com/hcraftsource.htm
-
- Posts: 1186
- Joined: Fri Dec 29, 2006 12:04 am