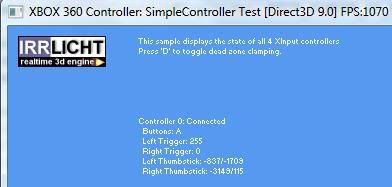
I've spent like a day on this. The picture above is from the simple test program based on the DirectX December SDK example of the same name. Of course, it's running inside irrlicht.

Here's what the example code looks like:
Code: Select all
#include <iostream>
#include "CIrrXbox360Controller.h"
// hide the console window
#pragma comment(linker, "/SUBSYSTEM:windows /ENTRY:mainCRTStartup")
int main()
{
// create xbox 360 controller class
irr::xb360controller::CIrrXbox360Controller xb360Controller;
// create device
IrrlichtDevice* device = createDevice(video::EDT_DIRECT3D9, core::dimension2d<s32>(800, 600));
if (device == 0)
return 1; // could not create selected driver.
video::IVideoDriver* driver = device->getVideoDriver();
scene::ISceneManager* smgr = device->getSceneManager();
gui::IGUIEnvironment* env = device->getGUIEnvironment();
driver->setTextureCreationFlag(video::ETCF_ALWAYS_32_BIT, true);
// add irrlicht logo
env->addImage(driver->getTexture("media/irrlichtlogoalpha.tga"),
core::position2d<s32>(10,10));
// add explanation text
gui::IGUIStaticText* textExplanation = env->addStaticText(
L"This sample displays the state of all 4 XInput controllers\nPress 'D' to toggle dead zone clamping.",
core::rect<s32>(130,10,500,70), false, true, 0, -1, false);
// add test value text
gui::IGUIStaticText* textTestValues = env->addStaticText(
L"Buttons: ",
core::rect<s32>(130,90,550,800), false, true, 0, -1, false);
video::SColor textColor(255,255,255,255);
textExplanation->setOverrideColor(textColor);
textTestValues->setOverrideColor(textColor);
// disable mouse cursor
device->getCursorControl()->setVisible(false);
int lastFPS = -1;
u32 dwResult = 0;
wchar_t sz[1024];
video::SColor background(0,85,153,240);
while(device->run())
if (device->isWindowActive())
{
driver->beginScene(true, true, background );
smgr->drawAll();
env->drawAll();
// Simply get the state of the controller from XInput.
dwResult = xb360Controller.getXInputState(0);
if(dwResult == ERROR_SUCCESS)
xb360Controller.setConnected(true);
else
xb360Controller.setConnected(false);
if(xb360Controller.isConnected())
{
WORD wButtons = xb360Controller.getButtons();
swprintf(sz,1024,
L"Controller %d: Connected\n"
L" Buttons: %s%s%s%s%s%s%s%s%s%s%s%s%s%s\n"
L" Left Trigger: %d\n"
L" Right Trigger: %d\n"
L" Left Thumbstick: %d/%d\n"
L" Right Thumbstick: %d/%d", 0,
(wButtons & XINPUT_GAMEPAD_DPAD_UP) ? L"DPAD_UP " : L"",
(wButtons & XINPUT_GAMEPAD_DPAD_DOWN) ? L"DPAD_DOWN " : L"",
(wButtons & XINPUT_GAMEPAD_DPAD_LEFT) ? L"DPAD_LEFT " : L"",
(wButtons & XINPUT_GAMEPAD_DPAD_RIGHT) ? L"DPAD_RIGHT " : L"",
(wButtons & XINPUT_GAMEPAD_START) ? L"START " : L"",
(wButtons & XINPUT_GAMEPAD_BACK) ? L"BACK " : L"",
(wButtons & XINPUT_GAMEPAD_LEFT_THUMB) ? L"LEFT_THUMB " : L"",
(wButtons & XINPUT_GAMEPAD_RIGHT_THUMB) ? L"RIGHT_THUMB " : L"",
(wButtons & XINPUT_GAMEPAD_LEFT_SHOULDER) ? L"LEFT_SHOULDER " : L"",
(wButtons & XINPUT_GAMEPAD_RIGHT_SHOULDER) ? L"RIGHT_SHOULDER " : L"",
(wButtons & XINPUT_GAMEPAD_A) ? L"A " : L"",
(wButtons & XINPUT_GAMEPAD_B) ? L"B " : L"",
(wButtons & XINPUT_GAMEPAD_X) ? L"X " : L"",
(wButtons & XINPUT_GAMEPAD_Y) ? L"Y " : L"",
xb360Controller.getLeftTrigger(),
xb360Controller.getRightTrigger(),
xb360Controller.getThumbLX(),
xb360Controller.getThumbLY(),
xb360Controller.getThumbRX(),
xb360Controller.getThumbRY() );
textTestValues->setText(sz);
}
else
{
swprintf(sz, 1024,
L"Controller %d: Not connected", 0 );
textTestValues->setText(sz);
}
driver->endScene();
// display frames per second in window title
int fps = driver->getFPS();
if (lastFPS != fps)
{
core::stringw str = L"XBOX 360 Controller: SimpleController Test [";
str += driver->getName();
str += "] FPS:";
str += fps;
device->setWindowCaption(str.c_str());
lastFPS = fps;
}
}
device->drop();
return 0;
}

TODO:
- rumble support (shouldn't be that hard to add)
- camera that uses the analog sticks for mouse movement
- bind the buttons to irrlicht keys without changing the irrlicht inner code

Update:
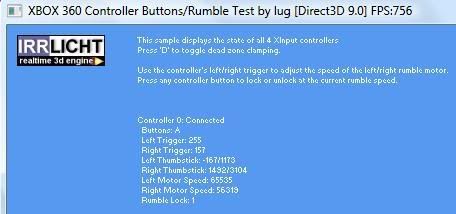
Rumble support is in!

Here's a win32 binary to try it out:
http://www.megaupload.com/?d=YDNXCUA0
Update 2:
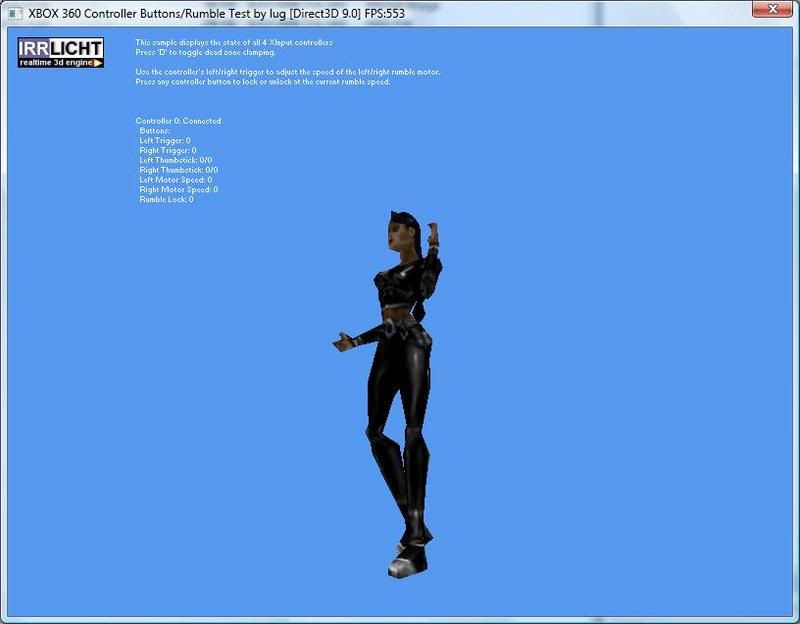
Analog controlled camera and character rotation working! Camera code based on the "Dead Easy 3rd Person Camera" from here:
http://irrlicht.sourceforge.net/phpBB2/ ... php?t=7977
The left thumb stick controls the character rotation. The right thumb stick controls the camera rotation. Right now, everything is too fast. I need to fine tune the math a bit.
Anyway, give it a whirl:
http://www.megaupload.com/?d=IBP2UZI0
Update 3:
Yay!

"A" button for attack:
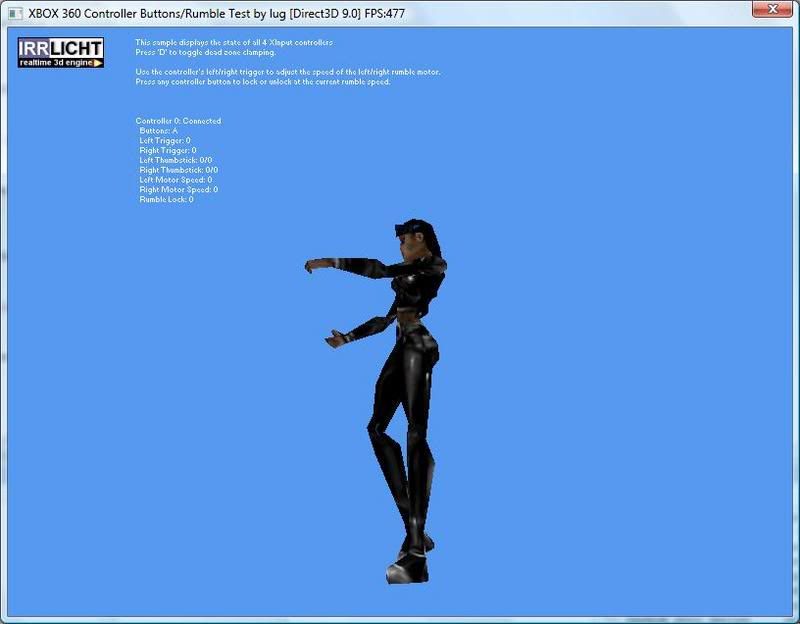
Left thumb button for crouch:
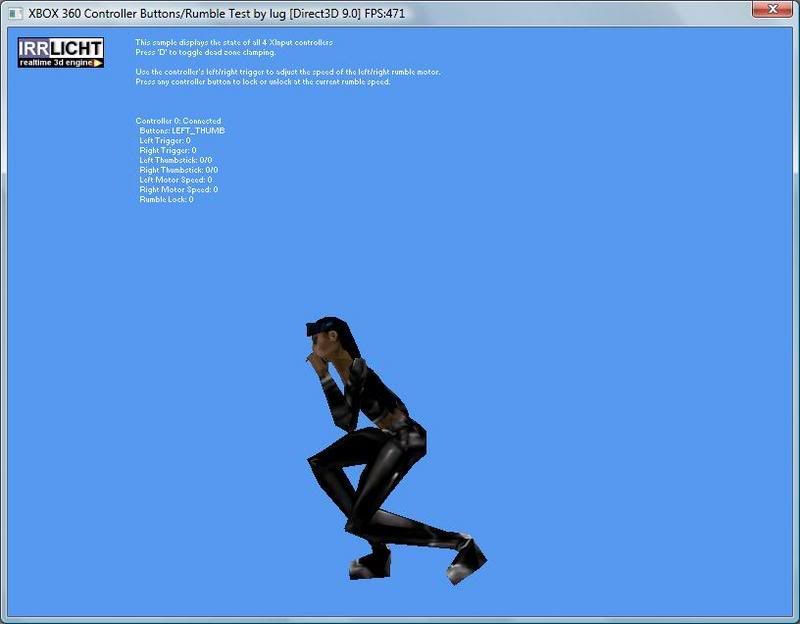
Left shoulder button for flip:
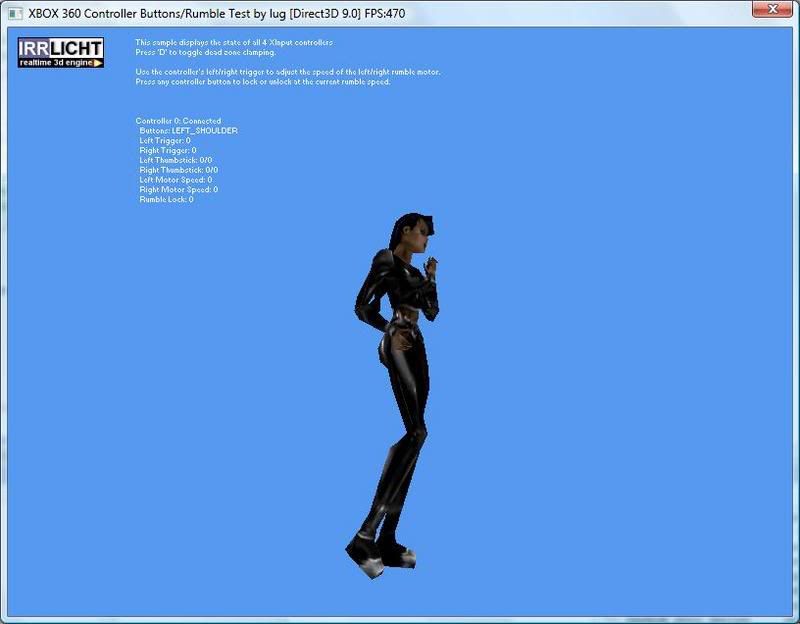
"B" button for jump:
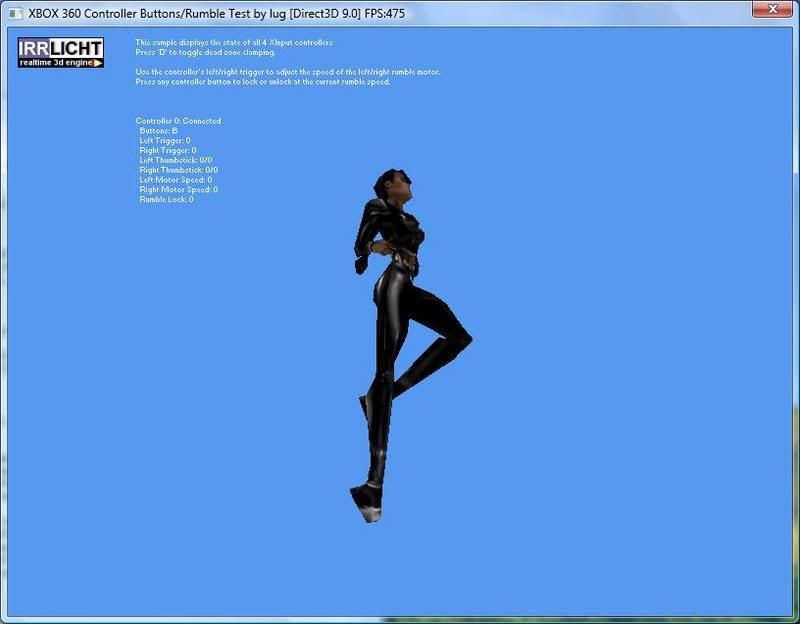
Right shoulder button for run:
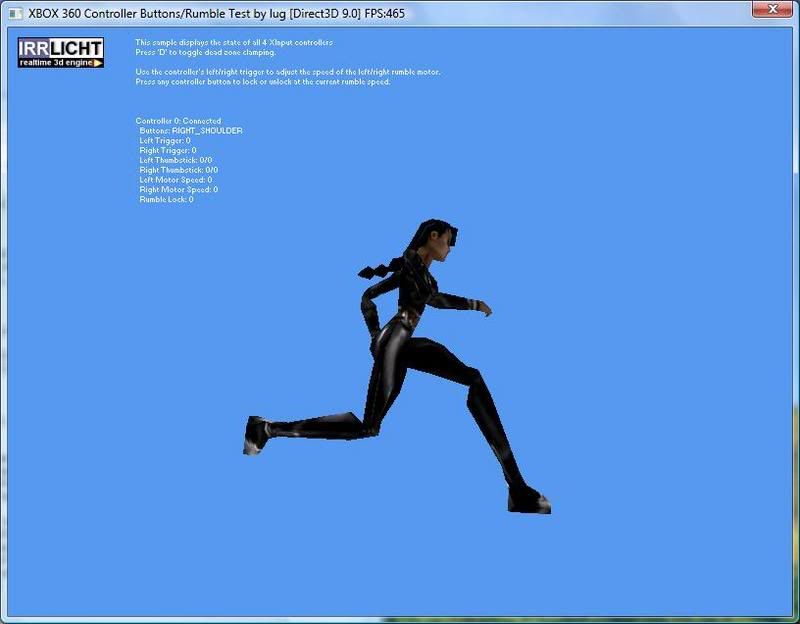
Update 4:
Start button to show window/Back button to close window:
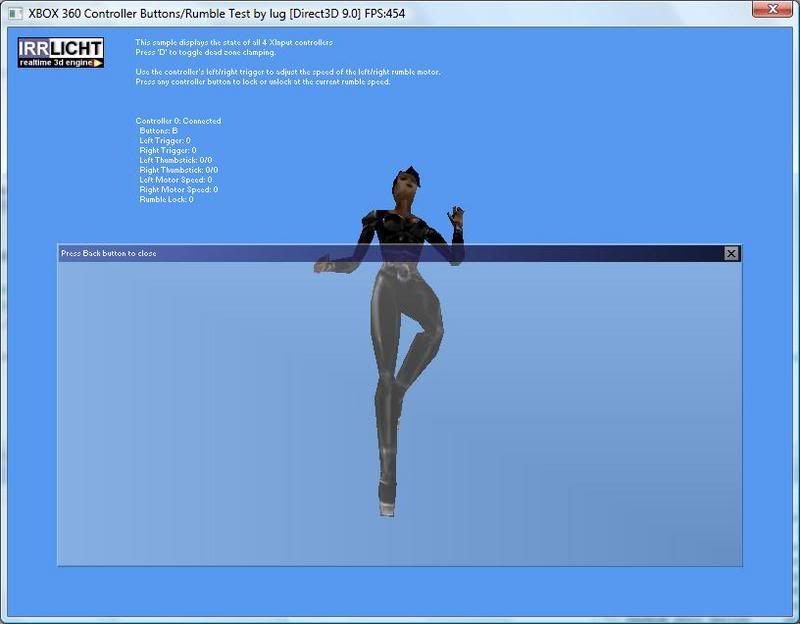
Download:
http://www.megaupload.com/?d=XOCEF9PI
Update 5:
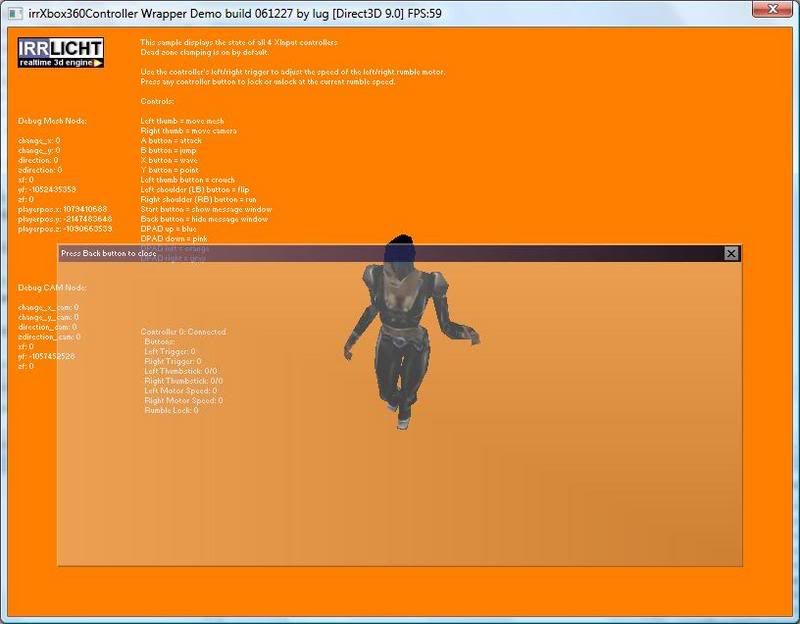
The thumb stick behaves a little bit now (try holding down in one direction for a smooth spin). Tapping the thumb stick makes it rotate like crazy.
Anyway, I've added control mappings to the instruction and some debug information. I've made the DPAD change the window color.

Download:
http://www.megaupload.com/?d=7JHKDL0A
Update 6:
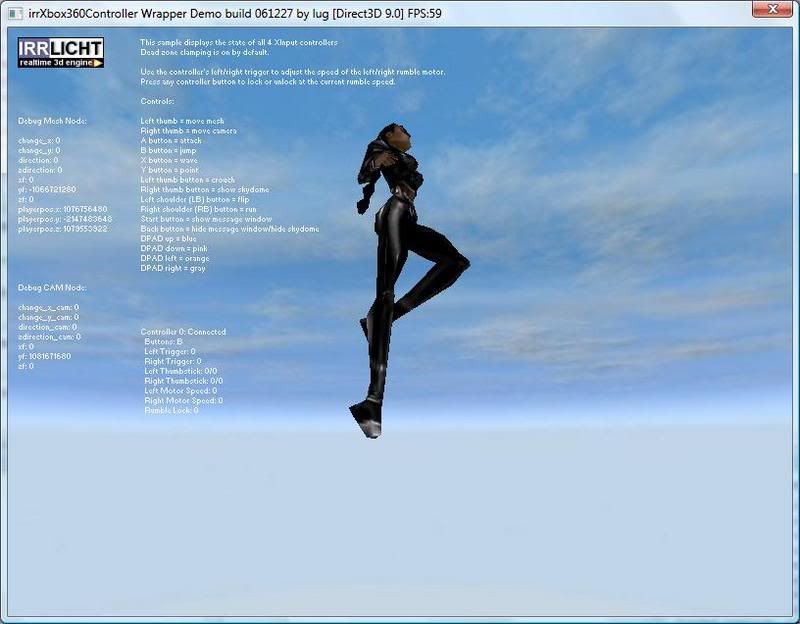
Thumb sticks "crazy fast" movement has been fixed! Also added a skydome as a reference point for orientation purposes.
Download (you know, megaupload is going to start charging me for uploading so frequently):
http://www.megaupload.com/?d=OGHXGQ6P
Update 7:
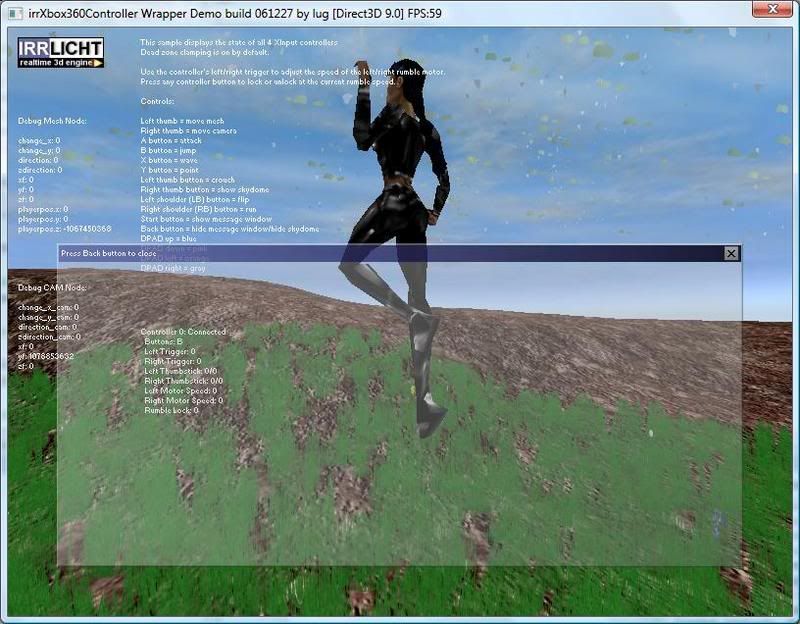
Now the mesh can move using the left thumb stick (disabled rotation of mesh though). Also added a terrain, bitplane's grass node with klasker's wind generator, and bitplane's cloud node to help with orientation.
Download:
http://www.megaupload.com/?d=EJNR8Q91