Hi,
so it's easy to add a cube or even sphere, but how do i add a simple plane? Basically, something like a billboard that doesn't rotate to the camera.
Thanks.
A plane node?
A plane node?
irrRenderer 1.0
Height2Normal v. 2.1 - convert height maps to normal maps
Step back! I have a void pointer, and I'm not afraid to use it!
Height2Normal v. 2.1 - convert height maps to normal maps
Step back! I have a void pointer, and I'm not afraid to use it!
Code: Select all
scene::SMesh* createPlaneMesh(f32 width = 1.f, f32 height = 1.f)
{
video::S3DVertex v0(0.f,0.f,0.f, 0.f,0.f,1.f,
video::SColor(255,255,255,255), 0.f,1.f);
video::S3DVertex v1(width,0.f,0.f, 0.f,0.f,1.f,
video::SColor(255,255,255,255), 1.f,1.f);
video::S3DVertex v2(width,height,0.f, 0.f,0.f,1.f,
video::SColor(255,255,255,255), 1.f,0.f);
video::S3DVertex v3(0.f,height,0.f, 0.f,0.f,1.f,
video::SColor(255,255,255,255), 0.f,0.f);
scene::CMeshBuffer<video::S3DVertex> *buffer =
new scene::CMeshBuffer<video::S3DVertex>;
buffer->Vertices.push_back(v0);
buffer->Vertices.push_back(v1);
buffer->Vertices.push_back(v2);
buffer->Vertices.push_back(v3);
buffer->Indices.push_back(0);
buffer->Indices.push_back(1);
buffer->Indices.push_back(2);
buffer->Indices.push_back(0);
buffer->Indices.push_back(2);
buffer->Indices.push_back(3);
buffer->recalculateBoundingBox();
scene::SMesh *mesh = new scene::SMesh;
mesh->addMeshBuffer(buffer);
buffer->drop();
mesh->recalculateBoundingBox();
return mesh;
}
Code: Select all
scene::ISceneNode* createPlaneSceneNode(scene::ISceneManager* smgr,
scene::ISceneNode *parent = NULL, s32 id = -1,
core::vector3d<f32> position = core::vector3d<f32>(0.f,0.f,0.f),
f32 width = 1.f, f32 height = 1.f)
{
video::S3DVertex v0(0.f,0.f,0.f, 0.f,0.f,1.f,
video::SColor(255,255,255,255), 0.f,1.f);
video::S3DVertex v1(width,0.f,0.f, 0.f,0.f,1.f,
video::SColor(255,255,255,255), 1.f,1.f);
video::S3DVertex v2(width,height,0.f, 0.f,0.f,1.f,
video::SColor(255,255,255,255), 1.f,0.f);
video::S3DVertex v3(0.f,height,0.f, 0.f,0.f,1.f,
video::SColor(255,255,255,255), 0.f,0.f);
scene::CMeshBuffer<video::S3DVertex> *buffer =
new scene::CMeshBuffer<video::S3DVertex>;
buffer->Vertices.push_back(v0);
buffer->Vertices.push_back(v1);
buffer->Vertices.push_back(v2);
buffer->Vertices.push_back(v3);
buffer->Indices.push_back(0);
buffer->Indices.push_back(1);
buffer->Indices.push_back(2);
buffer->Indices.push_back(0);
buffer->Indices.push_back(2);
buffer->Indices.push_back(3);
buffer->recalculateBoundingBox();
scene::SMesh *mesh = new scene::SMesh;
mesh->addMeshBuffer(buffer);
buffer->drop();
mesh->recalculateBoundingBox();
scene::ISceneNode *plane = smgr->addMeshSceneNode(mesh, parent, id);
mesh->drop();
plane->setPosition(position);
return plane;
}
Last edited by arras on Mon Mar 22, 2010 2:32 pm, edited 1 time in total.
Ah, nice, I was only looking in ISceneManager.
Thanks.
Thanks.
irrRenderer 1.0
Height2Normal v. 2.1 - convert height maps to normal maps
Step back! I have a void pointer, and I'm not afraid to use it!
Height2Normal v. 2.1 - convert height maps to normal maps
Step back! I have a void pointer, and I'm not afraid to use it!
-
- Posts: 1186
- Joined: Fri Dec 29, 2006 12:04 am
I tried hill plane mesh, it's a good idea for me. I did as guide in tutorial about special FX using addMeshSceneNode instead of addWaterSurfaceSceneNode, and got ugly render, plane was not rendered well. I don't know why?
My purpose is creating a plane mesh and then changing color of some tiles when needed. How can I solve this problem below and move next step?
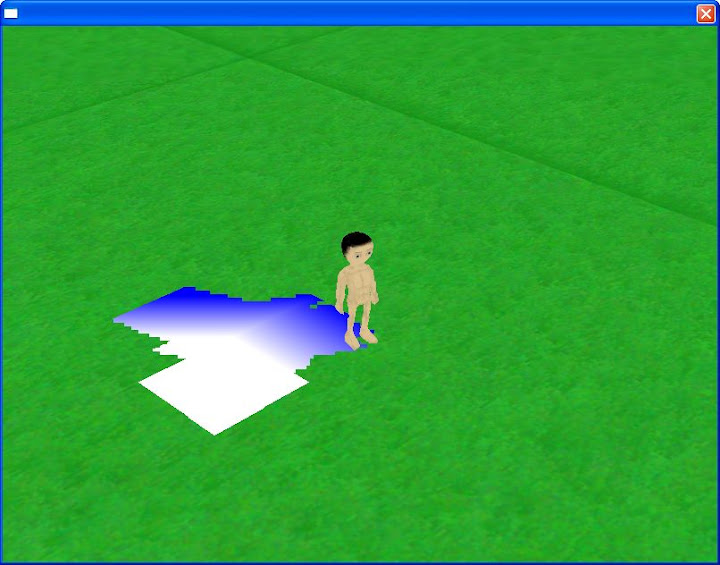
My purpose is creating a plane mesh and then changing color of some tiles when needed. How can I solve this problem below and move next step?
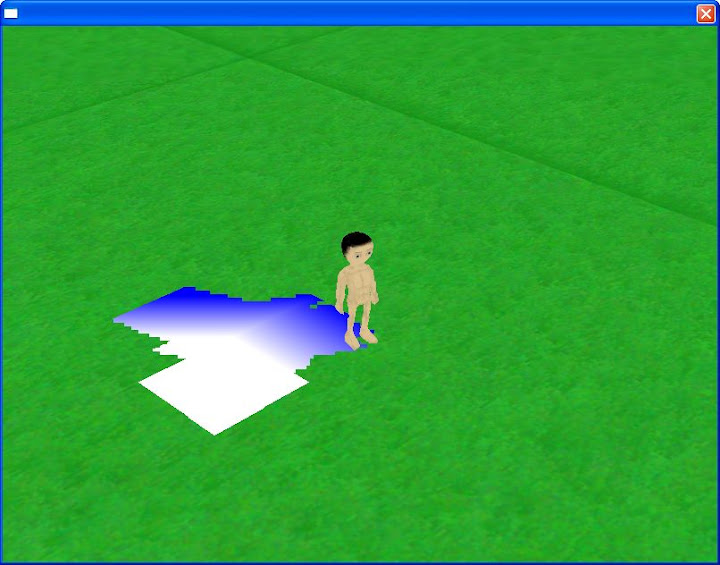
Last edited by vhson13 on Thu Apr 01, 2010 4:23 am, edited 1 time in total.