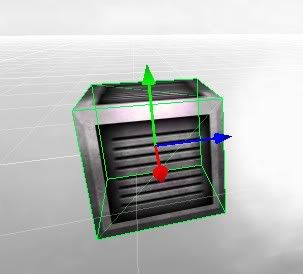
How to make those X, Y, Z axes visible when I translate or rotate a scene node?
How can I translate or rotate it according to the axis?
Could someone give me some example code?
I have encountered some problems when using draw3DLine function.Anthony wrote:at this page you can find tutorials
http://irrlicht.sourceforge.net/tutorials.html
next link is also good for starters
http://www.irrlicht3d.org/wiki/
for more advanced info there is
http://irrlicht.sourceforge.net/docu/index.html
these are the functions to change to pos and rot
ISceneNode::setPosition( vector3df( x, y, z ) );
ISceneNode::setRotation( vector3df( x, y, z ) );
to draw the axis
vector3df pos = ISceneNode::getPosition();
driver->draw3DLine( pos, pos + vector3df( 1, 0, 0 ), SColor( 255, 255, 0, 0 ) );
driver->draw3DLine( pos, pos + vector3df( 0, 1, 0 ), SColor( 255, 0, 255, 0 ) );
driver->draw3DLine( pos, pos + vector3df( 0, 0, 1 ), SColor( 255, 0, 0, 255 ) );
Code: Select all
SMaterial m; m.setTexture(0,0);
m.Lighting=false;
driver->setMaterial(m);
driver->setTransform(video::ETS_WORLD, core::matrix4());
driver->draw3DLine(SelectedNode->getAbsolutePosition(),vector3df(0,500,0),SColor(255,255,0,0));
Code: Select all
...getAbsolutePosition(), SelectedNode->getAbsolutePosition() + vector3df(0,500,0),...
Oops....thanks for pointing out that issue...Anthony wrote:the code looks fine too me.
One thing - propebly not the issue - butAlso make sure backface and frontface culling is off.Code: Select all
...getAbsolutePosition(), SelectedNode->getAbsolutePosition() + vector3df(0,500,0),...
(am a noobso hope I am helping here)
Code: Select all
_material.BackfaceCulling = true;
_material.FrontfaceCulling = false;
// wireframe is there too among others
Code: Select all
SMaterial m;
m.Lighting=false;
driver->setMaterial(m);
driver->setTransform(video::ETS_WORLD, core::matrix4());
driver->draw3DLine(vector3df(100,100,100),vector3df(0,500,0),SColor(255,255,0,0));
ICameraSceneNode *camera = smgr->addCameraSceneNodeFPS(0, 100.0f, 0.5f);
camera->setPosition(vector3df(0,0,0));
hybrid wrote:Oh, you must put the draw3DLine inside the render loop. This is not a scene node by itself, but needs to be called each frame
Code: Select all
#include <irrlicht.h>
using namespace irr;
using namespace core;
using namespace scene;
using namespace video;
using namespace io;
using namespace gui;
#ifdef _IRR_WINDOWS_
#pragma comment(lib, "Irrlicht.lib")
#pragma comment(linker, "/subsystem:windows /ENTRY:mainCRTStartup")
#endif
int main()
{
IrrlichtDevice *device =
createDevice( video::EDT_SOFTWARE, dimension2d<u32>(640, 480), 16,
false, false, false, 0);
if (!device)
return 1;
device->setWindowCaption(L"Hello World! - Irrlicht Engine Demo");
IVideoDriver* driver = device->getVideoDriver();
ISceneManager* smgr = device->getSceneManager();
IGUIEnvironment* guienv = device->getGUIEnvironment();
guienv->addStaticText(L"Hello World! This is the Irrlicht Software renderer!",
rect<s32>(10,10,260,22), true);
smgr->addCameraSceneNodeFPS(0, 100.0f, 0.5f);
while(device->run())
{
SMaterial material;
material.setFlag(video::EMF_LIGHTING, false);
material.setTexture(0, 0);
material.Lighting = false;
material.Wireframe=true;
driver->setMaterial(material);
driver->setTransform(video::ETS_WORLD, core::matrix4());
driver->draw3DLine(vector3df(0,0,0), vector3df(100,100,100), SColor(255,255,0,0));
driver->beginScene(true, true, SColor(255,100,101,140));
smgr->drawAll();
guienv->drawAll();
driver->endScene();
}
device->drop();
return 0;
}
Code: Select all
#include <irrlicht.h>
using namespace irr;
using namespace core;
using namespace scene;
using namespace video;
using namespace io;
using namespace gui;
#ifdef _IRR_WINDOWS_
#pragma comment(lib, "Irrlicht.lib")
// leave commented out to see some debug information: #pragma comment(linker, "/subsystem:windows /ENTRY:mainCRTStartup")
#endif
int main()
{
// Set it to a device that works, EDT_HYBRID maybe :P
IrrlichtDevice *device =
createDevice( video::EDT_OPENGL, dimension2d<u32>(640, 480), 16,
false, false, false, 0);
if (!device)
return 1;
device->setWindowCaption(L"Hello World! - Irrlicht Engine Demo");
IVideoDriver* driver = device->getVideoDriver();
ISceneManager* smgr = device->getSceneManager();
IGUIEnvironment* guienv = device->getGUIEnvironment();
guienv->addStaticText(L"Hello World! This is the Irrlicht Software renderer!",
rect<s32>(10,10,260,22), true);
ICameraSceneNode* camera = smgr->addCameraSceneNodeFPS(0, 100.0f, 0.5f);
// offset the camera a bit or you won't see the line either
camera->setPosition( vector3df( 10, 10, 10 ) );
camera->setTarget( vector3df( 0, 0, 0 ) );
while(device->run())
{
driver->beginScene(true, true, SColor(255,100,101,140));
// All rendering stuff happen between beginScene() and endScene()
SMaterial material;
material.Lighting = false;
driver->setMaterial(material);
// for this not needed: driver->setTransform(video::ETS_WORLD, core::matrix4());
driver->draw3DLine(vector3df(0,0,0), vector3df(-10,10,10), SColor(255,0,0,255));
smgr->drawAll();
guienv->drawAll();
driver->endScene();
}
device->drop();
return 0;
}