It disables the button on press and counts down till 0 and enables the button again.
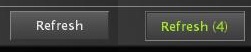
Code: Select all
//Create button
IGUIButton * button = new CGUICountDownButton(5, Environment, this, 1, core::rect<s32>(x, y, w, h));
button->setText(L"Some text");
button->drop();
Code: Select all
/**
* CGUICountDownButton.h
*
* Tank @ War Project
* February 2011
*/
#ifndef CGUICOUNTDOWNBUTTON_H_
#define CGUICOUNTDOWNBUTTON_H_
#include <irrlicht.h>
#include "CGUIButton.h"
namespace irr {
namespace gui {
class CGUICountDownButton: public CGUIButton {
protected:
irr::u32 timeDisabled;
irr::u32 countDownTime;
core::stringw originalText;
public:
CGUICountDownButton(u32 countDownTime, IGUIEnvironment* environment, IGUIElement* parent, s32 id, core::rect<s32> rectangle, bool noclip = false);
virtual ~CGUICountDownButton();
//! called if an event happened.
virtual bool OnEvent(const SEvent& event);
void setText(const wchar_t* text);
//! draws the element and its children
virtual void draw();
};
}
}
#endif /* CGUICOUNTDOWNBUTTON_H_ */
Code: Select all
// Tank @ War Client
#include "CGUICountDownButton.h"
#include "CGUIButton.cpp"
#include <stdio.h>
#include <time.h>
namespace irr {
namespace gui {
CGUICountDownButton::CGUICountDownButton(u32 countDownTime, IGUIEnvironment* environment, IGUIElement* parent, s32 id, core::rect<s32> rectangle, bool noclip) :
CGUIButton(environment, parent, id, rectangle, noclip) {
this->countDownTime = countDownTime;
setIsPushButton(true);
}
CGUICountDownButton::~CGUICountDownButton() {
}
bool CGUICountDownButton::OnEvent(const SEvent& event) {
if (CGUIButton::OnEvent(event) && isPressed() && isEnabled()) {
time_t now = time(NULL);
timeDisabled = (irr::u32) now;
setEnabled(false);
return true;
}
return false;
}
void CGUICountDownButton::setText(const wchar_t* text) {
originalText = text;
IGUIElement::setText(text);
}
void CGUICountDownButton::draw() {
time_t now = time(NULL);
u32 timeDiff = now - timeDisabled;
if (timeDiff < countDownTime && isPressed() && !isEnabled()) {
core::stringw text = originalText;
text += " (";
text += countDownTime - timeDiff;
text += ")";
IGUIElement::setText(text.c_str());
} else {
IGUIElement::setText(originalText.c_str());
setPressed(false);
setEnabled(true);
}
CGUIButton::draw();
}
}
}