This is really wierd, I mean really wierd and I hope I can explain what is happening.
Ok first of all this line does not work node->getMaterial(0).setTexture(1,"data/grenade/maps/grenadenrm.tga");
I get an error ||=== Build: Debug in mytests (compiler: GNU GCC Compiler) ===|
H:\Codeblock_Games\Irrlicht\Tests\03_MyTests\src\LoadMesh.cpp||In member function 'void LoadMesh::loadPiece(irr::scene::ISceneManager*, irr::video::IVideoDriver*, const wchar_t*)':|
H:\Codeblock_Games\Irrlicht\Tests\03_MyTests\src\LoadMesh.cpp|41|error: no matching function for call to 'irr::video::SMaterial::setTexture(int, const char [33])'|
H:\Codeblock_Games\Irrlicht\Tests\03_MyTests\src\LoadMesh.cpp|41|note: candidate is:|
H:\SDKs\irrlicht-1.8.1\irrlicht-1.8.1\include\SMaterial.h|482|note: void irr::video::SMaterial::setTexture(irr::u32, irr::video::ITexture*)|
H:\SDKs\irrlicht-1.8.1\irrlicht-1.8.1\include\SMaterial.h|482|note: no known conversion for argument 2 from 'const char [33]' to 'irr::video::ITexture*'|
||=== Build failed: 1 error(s), 0 warning(s) (0 minute(s), 0 second(s)) ===|
So let me now explain my set up.
I have a mesh loading class, as I said before. I have two functions in my meshloading class
I have a function called loadMesh and this is for loading all my meshes, and then I have a function called loadPiece. This function is a little different to load mesh as it loads a mesh and makes a camera also.
Because the line above would not work I added this line instead node->setMaterialTexture(1, driver->getTexture("data/grenade/maps/grenadenrm.tga"));
Now when I added that line to my loadPiece function it didn't really do anything.
When I added the same line to the loadMesh function suddenly the normalmap worked. Which is strange because I am not even calling that function.
Although I say it worked, but it is all deformed.
So then I think perhaps it is because irrlicht is loading the normalmap twice. So I renamed the line of the normalmap in the mtl file so it couldn't load, and then the normalmap wouldn't work at all.
In main I am setting up my class with
Code: Select all
LoadMesh grenade;
Code: Select all
grenade.loadPiece(smgr,driver,L"data/grenade/grenade.obj");
grenade.nodePosition(21.0f,79.3f,21.0f);
Then here is my full LoadMesh.cpp file. Notice I have put the line node->setMaterialTexture(1, driver->getTexture("data/grenade/maps/grenadenrm.tga")); in a function which isn't even being run.
And yet it kinda works. Something really wierd is going on here. I hope you understand what I am talking about. I say it kinda works because even though you can plainly see the normalmap in the picture, it is slightly deformed. Almost like it is something LOL. My head is gonna explode.
Code: Select all
#include "LoadMesh.h"
using namespace irr;
using namespace core;
using namespace video;
using namespace scene;
using namespace io;
using namespace gui;
LoadMesh::LoadMesh()
{
//constructor
}
void LoadMesh::loadMesh(ISceneManager* smgr,IVideoDriver* driver,const wchar_t* modelname)
{
mesh = smgr->getMesh(modelname);
node = smgr->addMeshSceneNode(mesh);
if (node)
{
node->setMaterialFlag(EMF_LIGHTING, true);
node->setPosition(core::vector3df(0,0,0));
node->addShadowVolumeSceneNode();
node->setMaterialTexture(1, driver->getTexture("data/grenade/maps/grenadenrm.tga"));
}
}
void LoadMesh::loadPiece(ISceneManager* smgr,IVideoDriver* driver,const wchar_t* modelname)
{
mesh = smgr->getMesh(modelname);
node = smgr->addMeshSceneNode(mesh);
if (node)
{
node->setMaterialFlag(EMF_LIGHTING, true);
node->setPosition(core::vector3df(0,0,0));
node->addShadowVolumeSceneNode();
node->setRotation(vector3df(-50,0,0));
camera = smgr->addCameraSceneNode(node,vector3df(0, 5, 5));
camera->bindTargetAndRotation(true);
camera->setTarget(node->getPosition());
}
}
void zoomCamera(ISceneManager* smgr)
{
// smgr->setActiveCamera(camera);
}
void LoadMesh::RotateMesh(float *rotation,const irr::f32 frameDeltaTime)
{
// *rotation-=50 * frameDeltaTime;
node->setRotation(core::vector3df(0,*rotation,0));
}
void LoadMesh::nodePosition(float x,float y,float z)
{
node->setPosition(vector3df(x,y,z));
}
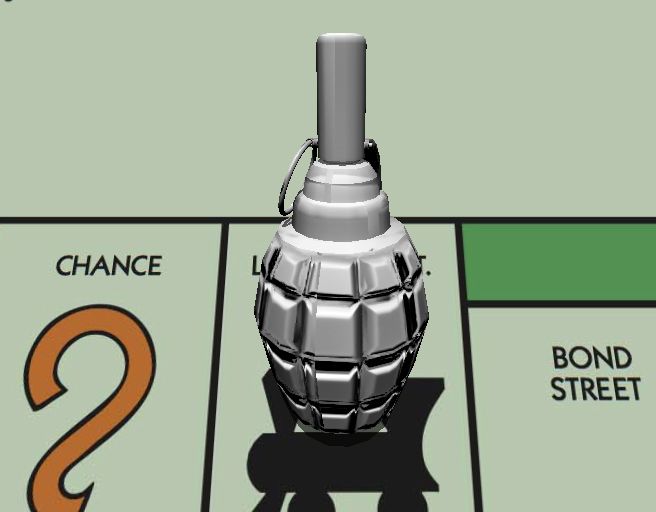