H all,
Normally with engines the 2D stuff is easy and the 3D stuff is harder, but I find I am having trouble showing a 2D image on the screen.
I have gone through tutorial 6 and it compiles and runs ok, but when I add the same code to my own project no 2D comes up.
The only difference between the tutorial and my project is that I have a movable 3D camara.
The other thing is that using the colour at (0,0) is ok, but it can produce hard edges.
In my previous engine I was able to load pngs which mean I can have soft edges because in a png you can have different levels of transparency.
Is there any way to use png sprites, or load pngs in irrlicht?
2D harder than 3D
Re: 2D harder than 3D
Are you calling your 2D functions before or after calling drawAll?
Irrlicht already supports PNGs, you just load them with getTexture and use draw2DImage like you would with any other image.
Irrlicht already supports PNGs, you just load them with getTexture and use draw2DImage like you would with any other image.
Re: 2D harder than 3D
Hi Juanpro,
I was calling the 2D functions before drawAll and after beginscene, but now I have put them after, and now 2D is working. Thanks.
Now I have write a class to simplify using 2D objects.
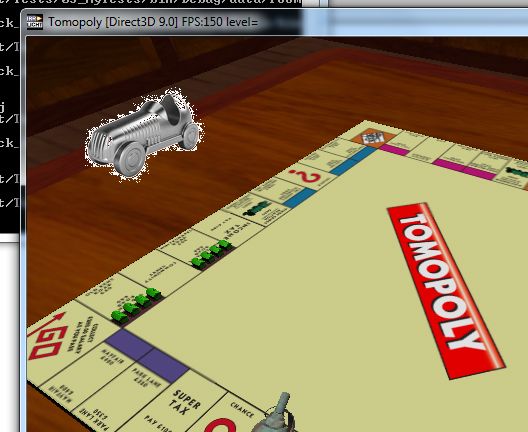
Now I have to decide what real screen dimensions I am going to use, as I don't want to do a pile of images to find out I can't resize them or anything LOL.
I wonder if you would be kind enough to show me how to load in a png using the gettexture command?
I was calling the 2D functions before drawAll and after beginscene, but now I have put them after, and now 2D is working. Thanks.
Now I have write a class to simplify using 2D objects.
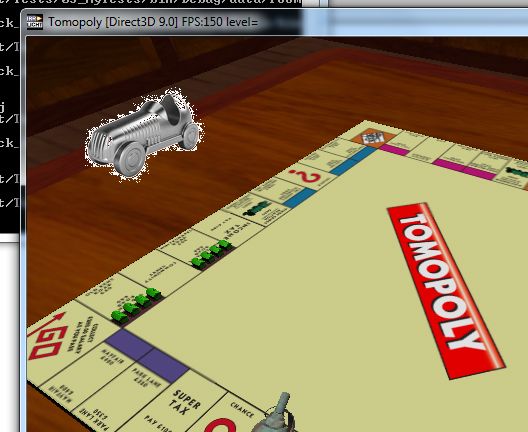
Now I have to decide what real screen dimensions I am going to use, as I don't want to do a pile of images to find out I can't resize them or anything LOL.
I wonder if you would be kind enough to show me how to load in a png using the gettexture command?
Re: 2D harder than 3D
getTexture should be able to load PNGs by default. You need to set the last parameter of draw2DImage to true to make it use the alpha channel.
Code: Select all
irr::video::ITexture* texture = driver->getTexture("image.png");
driver->draw2DImage(texture,destRect,sourceRect,0,0,true);
Re: 2D harder than 3D
Hi Juanpro,
Thanks I have been busy. Now you have given me that code I will try and get that working. However I have made a simple sprite loading class which I am pleased about.
Obviouslyy I want to make it better than this later by adding scale, rotation and stuff like that.
PS. just tried my sprite routine to load a png and it worked. Thanks Juanpro, you are a genius.
Sprite.h
Sprite.cpp
Thanks I have been busy. Now you have given me that code I will try and get that working. However I have made a simple sprite loading class which I am pleased about.
Obviouslyy I want to make it better than this later by adding scale, rotation and stuff like that.
PS. just tried my sprite routine to load a png and it worked. Thanks Juanpro, you are a genius.
Sprite.h
Code: Select all
#ifndef SPRITE_H
#define SPRITE_H
#include "irrlicht.h"
class Sprite
{
public:
// Sprite();
Sprite(irr::video::IVideoDriver *driver,const wchar_t* texturename,float x,float y);
irr::video::IVideoDriver * driver;
irr::video::ITexture * texture;
irr::core::rect<irr::s32> visible_area;
irr::core::vector2d<irr::s32> position;
irr::core::vector2d<irr::s32> center;
irr::core::vector2d<irr::f32> scale;
void Draw();
private:
};
Code: Select all
#include "Sprite.h"
using namespace irr;
using namespace core;
using namespace video;
Sprite::Sprite(IVideoDriver * driver,const wchar_t* texturename,float x,float y)
{
//ctor
this->driver = driver;
position = vector2d<s32>(x, y);
scale = vector2d<f32>(1.0f, 1.0f);
texture = driver->getTexture(texturename);
}
void Sprite::Draw()
{
driver->draw2DImage(texture, core::position2d<s32>(position),core::rect<s32>(0,0,130,97), 0,video::SColor(255,255,255,255), true);
}